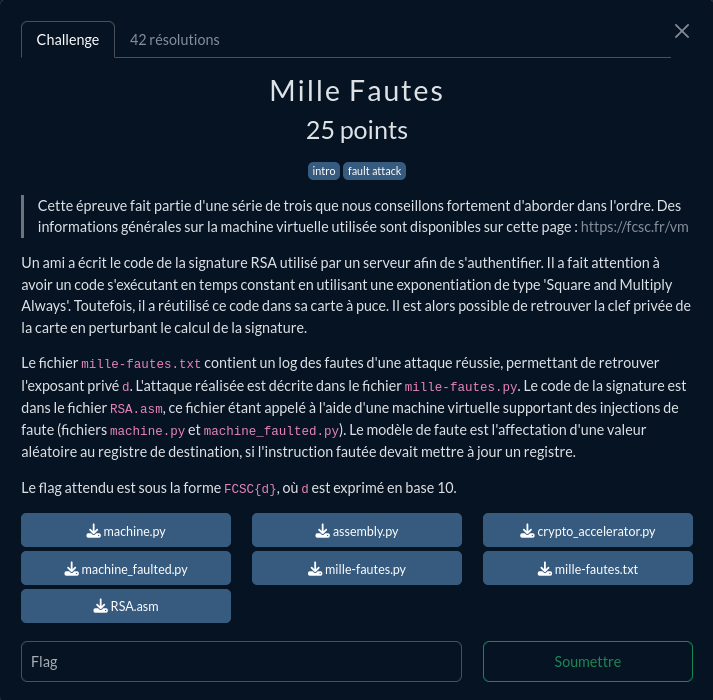
Introduction
Cet exercice illustre une attaque par fautes sur une implémentation RSA en temps constant (« Square and Multiply Always ») exécutée sur une carte à puce simulée. Bien que le code vise à empêcher les attaques par canaux cachés temporels, l’injection aléatoire de défauts dans le registre de destination révèle l’exposant privé d
bit à bit.
Contexte et fichiers (disponibles à la fin)
- RSA.asm : code assembleur de la fonction de signature RSA, utilisant la méthode « Square and Multiply Always ».
- machine.py et machine_faulted.py : simulateurs de la carte à puce, le second gérant l’injection de fautes.
- mille-fautes.py : script Python orchestrant l’attaque, interrogeant l’utilisateur pour l’index d’instruction fautée et loggant le succès (
True
) ou l’échec (False
). - mille-fautes.txt : journal de l’attaque, contenant 1024 lignes indiquant pour chaque bit si la signature est vérifiée malgré la faute.
Modèle de failles
Le simulateur injecte une valeur aléatoire dans le registre de destination (Rdst
) dès que le numéro d’instruction exécutée correspond à l’index spécifié. Ce défaut équivaut à commuter le bit de l’exposant :
- Si le bit original était 0, la faute n’affecte pas le résultat de la signature, donc la vérification reste
True
. - Si le bit original était 1, la faute perturbe la signature, la vérification échoue (
False
).
Extraction bit-à-bit
- Pour chaque bit
i
ded
, on exécute la signature en fautant l’instruction indexée par le journal. - On note le résultat de la vérification :
True
→ bit original = 0False
→ bit original = 1
- On reconstruit la chaîne binaire
b[0]…b[1023]
de l’exposant, oùb[i]
correspond au bit de poids faible à l’indicei
. - On inverse l’ordre de la chaîne (
b[1023]
devient bit de poids fort) pour obtenird
.
Reconstruction de l’exposant privé
- Lecture et interprétation de mille-fautes.txt pour générer la chaîne binaire.
- Conversion de la chaîne binaire inversée en entier
d
de 1024 bits. - Vérification : pour plusieurs messages
M
, on calcule la signatures = M^d mod N
et on vérifie ques^e ≡ M (mod N)
.
Résultat
L’exposant privé reconstruit est :
105242162543788743337252980031094540410286920689651093978118453037560701236944536116502819554965696785102170204949088553998761871348511118790932114247603701322088137236444818782787447039245943523349114909236634220118498903093045370684591251550088750568851495851919215974128144936137495423947422756508385773521
Le flag :
FCSC{105242162543788743337252980031094540410286920689651093978118453037560701236944536116502819554965696785102170204949088553998761871348511118790932114247603701322088137236444818782787447039245943523349114909236634220118498903093045370684591251550088750568851495851919215974128144936137495423947422756508385773521}
Annexes
machine.py :
from Crypto.Random.random import randrange
from crypto_accelerator import Fp_machine, ExactDivision, MillerRabin
import gmpy2
class Machine:
def __init__(self, code, e = 0, N = 0):
self.a = 0
self.b = 0
self.R0 = 0
self.R1 = 0
self.R2 = 0
self.R3 = 0
self.R4 = 0
self.R5 = 0
self.R6 = 0 # p
self.R7 = 0 # q
self.R8 = 0 # iq
self.R9 = 0 # dp
self.RA = 0 # dq
self.RB = e # e
self.exponent = 0 # d
self.module = N # n
self.lr = -1
self.pc = 0
self.tokenizeCode(code)
self.code_size = len(self.code)
self.end = False
self.error = False
self.nbInstruction = 0
self.FP_accelerator = None
self.instructionString = ""
def reset(self):
self.pc = 0
self.end = False
self.error = False
self.nbInstruction = 0
self.FP_accelerator = None
def tokenizeCode(self,s):
self.code = []
if len(s) % 4 != 0:
self.error = True
return
for i in range(len(s) // 4):
self.code.append(s[4*i:4*i+4])
def fetchInstruction(self):
self.instructionString = ""
c = self.code[self.pc]
opcode = int(c[:2], 16)
operand1 = 0
operand2 = 0
sop1 = ""
sop2 = ""
sdst = ""
# Case where the second byte contains only two operands
if (0 << 6) == opcode & (3 << 6) or (2 << 6) == opcode & (3 << 6):
operand1 = int(c[3], 16)
operand2 = int(c[2], 16)
self.dst = operand1
# Case where b15 b14 b13 b12 b11 b10 b9 b8 b7 b6 b5 b4 b3 b2 b1 b0 => b13 b12 b11 b10 b9 is the instruction, b8 b7 b6 is the op2, b5 b4 b3 is the op1, b2 b1 b0 is the dst
if (1 << 6) == opcode & (3 << 6):
t = int(c[1:4], 16)
self.dst, t = 7 & t, t >> 3
operand1, t = 7 & t, t >> 3
operand2 = 7 & t
opcode &= ((1 << 7) - 1) << 1
if 0 == operand1: self.a = self.R0
if 1 == operand1: self.a = self.R1
if 2 == operand1: self.a = self.R2
if 3 == operand1: self.a = self.R3
if 4 == operand1: self.a = self.R4
if 5 == operand1: self.a = self.R5
if 6 == operand1: self.a = self.R6
if 7 == operand1: self.a = self.R7
if 8 == operand1: self.a = self.R8
if 9 == operand1: self.a = self.R9
if 10 == operand1: self.a = self.RA
if 11 == operand1: self.a = self.RB
if 12 == operand1: self.a = self.exponent
if 13 == operand1: self.a = self.module
if 14 == operand1: self.a = self.lr
if 15 == operand1: self.a = self.pc
if 0 == operand2: self.b = self.R0
if 1 == operand2: self.b = self.R1
if 2 == operand2: self.b = self.R2
if 3 == operand2: self.b = self.R3
if 4 == operand2: self.b = self.R4
if 5 == operand2: self.b = self.R5
if 6 == operand2: self.b = self.R6
if 7 == operand2: self.b = self.R7
if 8 == operand2: self.b = self.R8
if 9 == operand2: self.b = self.R9
if 10 == operand2: self.b = self.RA
if 11 == operand2: self.b = self.RB
if 12 == operand2: self.b = self.exponent
if 13 == operand2: self.b = self.module
if 14 == operand2: self.b = self.lr
if 15 == operand2: self.b = self.pc
sop1 = "R" + f"{operand1:01X}"
sop2 = "R" + f"{operand2:01X}"
sdst = "R" + f"{self.dst:01X}"
# Case where the instruction is on 4 bytes
if (2 << 6) == opcode & (3 << 6) :
self.pc += 1
self.b = int(self.code[self.pc], 16)
opcode = opcode & ((1 << 7)-1)
sop2 = f"{hex(self.b)}"
# Case where the second byte represents an 8-bit value
if (3 << 6) == opcode & (3 << 6):
self.b = int(c[2] + c[3], 16)
sop2 = f"{hex(self.b)}"
self.instruction = False
if 0 == opcode: self.instruction = self.move ; self.instructionString += "MOV " + ", ".join([sdst, sop2])
if (0 + (2 << 6)) == opcode: self.instruction = self.move ; self.instructionString += "MOV " + ", ".join([sdst, sop2])
if (0 + (1 << 6)) == opcode: self.instruction = self.log_and ; self.instructionString += "AND " + ", ".join([sdst, sop1, sop2])
if (2 + (1 << 6)) == opcode: self.instruction = self.log_or ; self.instructionString += "OR " + ", ".join([sdst, sop1, sop2])
if (4 + (1 << 6)) == opcode: self.instruction = self.log_xor ; self.instructionString += "XOR " + ", ".join([sdst, sop1, sop2])
if (6 + (1 << 6)) == opcode: self.instruction = self.shiftL ; self.instructionString += "SLL " + ", ".join([sdst, sop1, sop2])
if (8 + (1 << 6)) == opcode: self.instruction = self.shiftR ; self.instructionString += "SRL " + ", ".join([sdst, sop1, sop2])
if 1 == opcode: self.instruction = self.bit_len ; self.instructionString += "BTL " + ", ".join([sdst, sop2])
if (10 + (1 << 6)) == opcode: self.instruction = self.add ; self.instructionString += "ADD " + ", ".join([sdst, sop1, sop2])
if (12 + (1 << 6)) == opcode: self.instruction = self.sub ; self.instructionString += "SUB " + ", ".join([sdst, sop1, sop2])
if (14 + (1 << 6)) == opcode: self.instruction = self.mul ; self.instructionString += "MUL " + ", ".join([sdst, sop1, sop2])
if (16 + (1 << 6)) == opcode: self.instruction = self.div ; self.instructionString += "DIV " + ", ".join([sdst, sop1, sop2])
if 2 == opcode: self.instruction = self.mod ; self.instructionString += "MOD " + ", ".join([sdst, sop2])
if 3 == opcode: self.instruction = self.pow ; self.instructionString += "POW " + ", ".join([sdst, sop2])
if (18 + (1 << 6)) == opcode: self.instruction = self.gcd ; self.instructionString += "GCD " + ", ".join([sdst, sop1, sop2])
if 4 == opcode: self.instruction = self.invert ; self.instructionString += "INV " + ", ".join([sdst, sop2])
if 5 == opcode: self.instruction = self.random ; self.instructionString += "RND " + ", ".join([sdst])
if 6 == opcode: self.instruction = self.cmp ; self.instructionString += "CMP " + ", ".join([sop1, sop2])
if 24 == opcode: self.instruction = self.init_FP_accelerator ; self.instructionString += "FP " + ", ".join([sop1, sop2])
if (24 + (1 << 6)) == opcode: self.instruction = self.init_FP_accelerator_withRR ; self.instructionString += "FPRR " + ", ".join([sdst, sop1, sop2])
if 26 == opcode: self.instruction = self.MM1 ; self.instructionString += "MM1 " + ", ".join([sdst, sop2])
if (26 + (1 << 6)) == opcode: self.instruction = self.MM ; self.instructionString += "MM " + ", ".join([sdst, sop1, sop2])
if 28 == opcode: self.instruction = self.movRR ; self.instructionString += "MOVRR " + ", ".join([sdst])
if (28 + (1 << 6)) == opcode: self.instruction = self.ExactDiv ; self.instructionString += "EDIV " + ", ".join([sdst, sop1, sop2])
if 25 == opcode: self.instruction = self.MontgomeryPow ; self.instructionString += "MPOW " + ", ".join([sdst, sop2])
if 27 == opcode: self.instruction = self.MillerRabin ; self.instructionString += "MR " + ", ".join([sop1])
# relative jumps have an odd opcode
if 7 == opcode or ((3 << 6) + 7) == opcode: self.instruction = self.jz_rel ; self.instructionString += "JZR " + sop2
if 8 == opcode or ((2 << 6) + 8) == opcode: self.instruction = self.jz_abs ; self.instructionString += "JZA " + sop2
if 9 == opcode or ((3 << 6) + 9) == opcode: self.instruction = self.jnz_rel ; self.instructionString += "JNZR " + sop2
if 10 == opcode or ((2 << 6) + 10) == opcode: self.instruction = self.jnz_abs ; self.instructionString += "JNZA " + sop2
if 11 == opcode or ((3 << 6) + 11) == opcode: self.instruction = self.jc_rel ; self.instructionString += "JCR " + sop2
if 12 == opcode or ((2 << 6) + 12) == opcode: self.instruction = self.jc_abs ; self.instructionString += "JCA " + sop2
if 13 == opcode or ((3 << 6) + 13) == opcode: self.instruction = self.jnc_rel ; self.instructionString += "JNCR " + sop2
if 14 == opcode or ((2 << 6) + 14) == opcode: self.instruction = self.jnc_abs ; self.instructionString += "JNCA " + sop2
if 15 == opcode or ((3 << 6) + 15) == opcode: self.instruction = self.j_rel ; self.instructionString += "JR " + sop2
if 16 == opcode or ((2 << 6) + 16) == opcode: self.instruction = self.j_abs ; self.instructionString += "JA " + sop2
if 17 == opcode or ((3 << 6) + 17) == opcode: self.instruction = self.call_rel ; self.instructionString += "CR " + sop2
if 18 == opcode or ((2 << 6) + 18) == opcode: self.instruction = self.call_abs ; self.instructionString += "CA " + sop2
if 19 == opcode: self.instruction = self.ret ; self.instructionString += "RET"
if 20 == opcode: self.instruction = self.stop ; self.instructionString += "STP"
if 21 == opcode: self.instruction = self.movc ; self.instructionString += "MOVC " + ",".join([sop1, sop2])
if 22 == opcode: self.instruction = self.movcb ; self.instructionString += "MOVCB " + sop1
if 23 == opcode: self.instruction = self.movcw ; self.instructionString += "MOVCW " + sop1
self.pc += 1
if not self.instruction:
self.error = True
def executeInstruction(self):
if not self.error:
self.instruction()
def runCode(self):
while True:
t = self.pc
self.fetchInstruction()
self.executeInstruction()
self.nbInstruction += 1
if self.end or self.error:
return self
if self.pc >= self.code_size or self.pc < 0 or self.nbInstruction > (1 << 16):
self.end = True
self.error = True
return self
def printState(self):
print("==" * 32)
print("")
print(self.instructionString)
print("")
print(f"a = {self.a}")
print(f"b = {self.b}")
print(f"R0 = {self.R0}")
print(f"R1 = {self.R1}")
print(f"R2 = {self.R2}")
print(f"R3 = {self.R3}")
print(f"R4 = {self.R4}")
print(f"R5 = {self.R5}")
print(f"R6 = {self.R6}")
print(f"R7 = {self.R7}")
print(f"R8 = {self.R8}")
print(f"R9 = {self.R9}")
print(f"RA = {self.RA}")
print(f"RB = {self.RB}")
print(f"exponent = {self.exponent}")
print(f"module = {self.module}")
print(f"lr = {self.lr}")
print(f"pc = {self.pc}")
print(f"error = {self.error}")
if self.FP_accelerator:
self.FP_accelerator.display()
def debugCode(self):
print("entering debug")
while True:
t = self.pc
self.fetchInstruction()
self.executeInstruction()
self.printState()
self.nbInstruction += 1
if self.end or self.error:
return self
if self.pc >= self.code_size or self.pc < 0 or self.nbInstruction > (1 << 16):
self.end = True
self.error = True
return self
def finalize_move(self):
if 0 == self.dst: self.R0 = self.a
if 1 == self.dst: self.R1 = self.a
if 2 == self.dst: self.R2 = self.a
if 3 == self.dst: self.R3 = self.a
if 4 == self.dst: self.R4 = self.a
if 5 == self.dst: self.R5 = self.a
if 6 == self.dst: self.R6 = self.a
if 7 == self.dst: self.R7 = self.a
if 8 == self.dst: self.R8 = self.a
if 9 == self.dst: self.R9 = self.a
if 10 == self.dst: self.RA = self.a
if 11 == self.dst: self.RB = self.a
if 12 == self.dst: self.exponent = self.a
if 13 == self.dst: self.module = self.a
if 14 == self.dst: self.lr = self.a
if 15 == self.dst: self.pc = self.a
def move(self):
self.a = self.b
self.finalize_move()
def log_and(self):
self.a = self.a & self.b
self.finalize_move()
def log_or(self):
self.a = self.a | self.b
self.finalize_move()
def log_xor(self):
self.a = self.a ^ self.b
self.finalize_move()
def shiftL(self):
self.a = self.a << self.b
self.finalize_move()
def shiftR(self):
self.a = self.a >> self.b
self.finalize_move()
def bit_len(self):
self.a = self.b.bit_length()
self.finalize_move()
def add(self):
self.a = self.a + self.b
self.finalize_move()
def sub(self):
self.C = (self.a >= self.b)
self.Z = (self.a == self.b)
self.a = self.a - self.b
self.finalize_move()
def mul(self):
self.Z = (self.a == 0 or self.b == 0)
self.a = self.a * self.b
self.finalize_move()
def div(self):
if self.b != 0:
self.a = self.a // self.b
self.finalize_move()
else:
self.error = True
def mod(self):
if self.module != 0:
self.a = self.b % self.module
self.finalize_move()
else:
self.error = True
def pow(self):
if self.module != 0:
self.a = int(gmpy2.powmod(self.b, self.exponent, self.module))
self.Z = (self.a == 0)
self.finalize_move()
else:
self.error = True
def gcd(self):
self.a = int(gmpy2.gcd(self.a, self.b))
self.finalize_move()
def invert(self):
if 1 == gmpy2.gcd(self.b, self.module) and (self.module != 0):
self.a = int(gmpy2.invert(self.b, self.module))
self.finalize_move()
else:
self.error = True
def random(self):
if 0 == self.a:
self.error = True
return
self.a = randrange(1 << self.a)
self.finalize_move()
def cmp(self):
self.C = (self.a >= self.b)
self.Z = (self.a == self.b)
def jz_rel(self):
if self.Z:
if self.b < (1 << 7):
self.pc += self.b
else:
self.pc -= (1 << 8) - self.b
def jnz_rel(self):
if not self.Z:
if self.b < (1 << 7):
self.pc += self.b
else:
self.pc -= (1 << 8) - self.b
def jc_rel(self):
if self.C:
if self.b < (1 << 7):
self.pc += self.b
else:
self.pc -= (1 << 8) - self.b
def jnc_rel(self):
if not self.C:
if self.b < (1 << 7):
self.pc += self.b
else:
self.pc -= (1 << 8) - self.b
def jz_abs(self):
if self.Z:
self.pc = self.b
def jnz_abs(self):
if not self.Z:
self.pc = self.b
def jc_abs(self):
if self.C:
self.pc = self.b
def jnc_abs(self):
if not self.C:
self.pc = self.b
def j_rel(self):
if self.b < (1 << 7):
self.pc += self.b
else:
self.pc -= (1 << 8) - self.b
def j_abs(self):
self.pc = self.b
def call_rel(self):
self.lr = self.pc
if self.b < (1 << 7):
self.pc += self.b
else:
self.pc -= (1 << 8) - self.b
def call_abs(self):
self.lr = self.pc
self.pc = self.b
def ret(self):
self.pc = self.lr
def stop(self):
self.end = True
def movcw(self):
c= self.code[self.a]
self.a = int(c[0:4],16)
self.finalize_move()
def movc(self):
t = 0
for i in range(self.b):
c = self.code[self.a+i]
t <<= 16
t ^= int(c[0:4], 16)
self.a = t
self.finalize_move()
def init_FP_accelerator(self):
if (0 != self.b) or (0 != self.a & 1):
self.FP_accelerator = Fp_machine(self.a, self.b)
else:
self.error = True
def init_FP_accelerator_withRR(self):
if (0 != self.b) or (0 != self.a & 1):
self.FP_accelerator = Fp_machine(self.a, self.b, self.dst)
else:
self.error = True
def movRR(self):
if self.FP_accelerator:
self.a = self.FP_accelerator.RR
self.finalize_move()
else:
self.error = True
def MM1(self):
if self.FP_accelerator:
self.a = self.FP_accelerator.MM(self.b, 1)
self.finalize_move()
else:
self.error = True
def MM(self):
if self.FP_accelerator:
self.a = self.FP_accelerator.MM(self.a, self.b)
self.finalize_move()
else:
self.error = True
def MontgomeryPow(self):
if self.FP_accelerator:
self.a = self.FP_accelerator.MPow(self.b, self.exponent)
self.finalize_move()
else:
self.error = True
def ExactDiv(self):
if self.b != 0 and 0 == (self.a%self.b):
self.a = ExactDivision(self.a, self.b)
self.finalize_move()
else:
self.error = True
def MillerRabin(self):
self.Z = MillerRabin(self.a)
assembly.py
import re
import sys
def assembly(asm):
res = ''
labels = {}
refs = []
for line in asm:
if not re.search(r'\w', line):
continue
if re.search(r'^\s*$', line):
continue
if re.search(r'^\s*;', line):
continue
if re.search(r'\W*([A-Za-z]\w*):', line):
b = re.findall(r'\W*([A-Za-z]\w*):',line)
labels[b[0]] = len(res) >> 2
continue
if re.search(r'\.word\b', line):
b = re.split(r'\.word\b', line)[1]
b = re.split(r',', b)
for i in range(len(b)):
if re.search(r'=[A-Za-z]\w*', b[i]):
refs.append(re.split(r'=', b[i])[1])
res += '(XX)'
continue
if re.search(r'0x', b[i]):
t = int(b[i], 16)
else:
t = int(b[i])
if t < (1<<16):
res += f"{t:04x}"
else:
print(f"{t} is not a word")
continue
opcode = 'FFFF'
if re.search(r'\bMOV\b', line):
b = re.findall(r'\bMOV\W*R([0-9A-Fa-f]),\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
opcode = '00' + b[0][1] + b[0][0]
b = re.findall(r'\bMOV\W*R([0-9A-Fa-f]),\s*#([0-9]+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][1])
if t < (1 << 16):
opcode = '800' + b[0][0] + f"{t:04x}"
b = re.findall(r'\bMOV\W*R([0-9A-Fa-f]),\s*#0x([0-9A-Fa-f]+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][1], 16)
if t < (1 << 16):
opcode = '800' + b[0][0] + f"{t:04x}"
b = re.findall(r'\bMOV\W*R([0-9A-Fa-f]),\s*=([A-Za-z]\w*)\s*($|;)',line)
if 0 < len(b):
refs.append(b[0][1])
opcode = '800' + b[0][0] + "(XX)"
if re.search(r'\bAND\b', line):
b = re.findall(r'\bAND\W*R([0-7]),\W*R([0-7]),\W*R([0-7])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16) + (int(b[0][1], 16) << 3) + (int(b[0][2], 16) << 6)
t += 0x4000
opcode = f"{t:04x}"
if re.search(r'\bOR\b', line):
b = re.findall(r'\bOR\W*R([0-7]),\W*R([0-7]),\W*R([0-7])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16) + (int(b[0][1], 16) << 3) + (int(b[0][2], 16) << 6)
t += 0x4200
opcode = f"{t:04x}"
if re.search(r'\bXOR\b', line):
b = re.findall(r'\bXOR\W*R([0-7]),\W*R([0-7]),\W*R([0-7])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16) + (int(b[0][1], 16) << 3) + (int(b[0][2], 16) << 6)
t += 0x4400
opcode = f"{t:04x}"
if re.search(r'\bSRL\b', line):
b = re.findall(r'\bSRL\W*R([0-7]),\W*R([0-7]),\W*R([0-7])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16) + (int(b[0][1], 16) << 3) + (int(b[0][2], 16) << 6)
t += 0x4800
opcode = f"{t:04x}"
if re.search(r'\bSLL\b', line):
b = re.findall(r'\bSLL\W*R([0-7]),\W*R([0-7]),\W*R([0-7])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16) + (int(b[0][1], 16) << 3) + (int(b[0][2], 16) << 6)
t += 0x4600
opcode = f"{t:04x}"
if re.search(r'\bBTL\b', line):
b = re.findall(r'\bBTL\W*R([0-9A-Fa-f]),\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16) + (int(b[0][1], 16) << 4)
t += 0x0100
opcode = f"{t:04x}"
if re.search(r'\bADD\b', line):
b = re.findall(r'\bADD\W*R([0-7]),\W*R([0-7]),\W*R([0-7])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16) + (int(b[0][1], 16) << 3) + (int(b[0][2], 16) << 6)
t += 0x4A00
opcode = f"{t:04x}"
if re.search(r'\bSUB\b', line):
b = re.findall(r'\bSUB\W*R([0-7]),\W*R([0-7]),\W*R([0-7])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16) + (int(b[0][1], 16) << 3) + (int(b[0][2], 16) << 6)
t += 0x4C00
opcode = f"{t:04x}"
if re.search(r'\bMUL\b', line):
b = re.findall(r'\bMUL\W*R([0-7]),\W*R([0-7]),\W*R([0-7])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16) + (int(b[0][1], 16) << 3) + (int(b[0][2], 16) << 6)
t += 0x4E00
opcode = f"{t:04x}"
if re.search(r'\bDIV\b', line):
b = re.findall(r'\bDIV\W*R([0-7]),\W*R([0-7]),\W*R([0-7])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16) + (int(b[0][1], 16) << 3) + (int(b[0][2], 16) << 6)
t += 0x5000
opcode = f"{t:04x}"
if re.search(r'\bMOD\b', line):
b = re.findall(r'\bMOD\W*R([0-9A-Fa-f]),\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16) + (int(b[0][1], 16) << 4)
t += 0x0200
opcode = f"{t:04x}"
if re.search(r'\bPOW\b', line):
b = re.findall(r'\bPOW\W*R([0-9A-Fa-f]),\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16) + (int(b[0][1], 16) << 4)
t += 0x0300
opcode = f"{t:04x}"
if re.search(r'\bGCD\b', line):
b = re.findall(r'\bGCD\W*R([0-7]),\W*R([0-7]),\W*R([0-7])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16) + (int(b[0][1], 16) << 3) + (int(b[0][2], 16) << 6)
t += 0x5200
opcode = f"{t:04x}"
if re.search(r'\bINV\b', line):
b = re.findall(r'\bINV\W*R([0-9A-Fa-f]),\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16) + (int(b[0][1], 16) << 4)
t += 0x0400
opcode = f"{t:04x}"
if re.search(r'\bRND\b', line):
b = re.findall(r'\bRND\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16)
t += 0x0500
opcode = f"{t:04x}"
if re.search(r'\bCMP\b', line):
b = re.findall(r'\bCMP\W*R([0-9A-Fa-f]),\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16) + (int(b[0][1], 16) << 4)
t += 0x0600
opcode = f"{t:04x}"
if re.search(r'\bMOVC\b', line):
b = re.findall(r'\bMOVC\W*R([0-9A-Fa-f]),\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16) + (int(b[0][1], 16) << 4)
t += (21) << 8
opcode = f"{t:04x}"
if re.search(r'\bMOVCW\b', line):
b = re.findall(r'\bMOVCW\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16)
t += (23) << 8
opcode = f"{t:04x}"
if re.search(r'\bJZR\b', line):
b = re.findall(r'\bJZR\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = (int(b[0][0], 16) << 4)
t += 0x0700
opcode = f"{t:04x}"
c = re.findall(r'\bJZR\s*([A-Za-z]\w*)\s*($|;)',line) #Use a second variable so that `Ri` is not considered as a label
if 0 == len(b) and 0 < len(c):
refs.append(c[0][0])
opcode = 'C7()'
b = re.findall(r'\bJZR\s*\+(\d+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0])
if t < (1 << 7):
t += 0xC700
opcode = f"{t:04x}"
b = re.findall(r'\bJZR\s*-(\d+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0])
if t <= (1 << 7) and 0 < t:
t = 256 - t
t += 0xC700
opcode = f"{t:04x}"
if re.search(r'\bJZA\b', line):
b = re.findall(r'\bJZA\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = (int(b[0][0], 16) << 4)
t += 0x0800
opcode = f"{t:04x}"
c = re.findall(r'\bJZA\s*([A-Za-z]\w*)\s*($|;)',line)
if 0 == len(b) and 0 < len(c):
refs.append(c[0][0])
opcode = '8800(XX)'
b = re.findall(r'\bJZA\s*#([0-9]+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0])
if t < (1 << 16):
opcode = '8800'+f"{t:04x}"
b = re.findall(r'\bJZA\s*#0x([0-9A-Fa-f]+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16)
if t < (1 << 16):
opcode = '8800'+f"{t:04x}"
if re.search(r'\bJNZR\b', line):
b = re.findall(r'\bJNZR\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = (int(b[0][0], 16) << 4)
t += 0x0900
opcode = f"{t:04x}"
c = re.findall(r'\bJNZR\s*([A-Za-z]\w*)\s*($|;)',line)
if 0 == len(b) and 0 < len(c):
refs.append(c[0][0])
opcode = 'C9()'
b = re.findall(r'\bJNZR\s*\+(\d+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0])
if t < (1 << 7):
t += 0xC900
opcode = f"{t:04x}"
b = re.findall(r'\bJNZR\s*-(\d+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0])
if t <= (1 << 7) and 0 < t:
t = 256 - t
t += 0xC900
opcode = f"{t:04x}"
if re.search(r'\bJNZA\b', line):
b = re.findall(r'\bJNZA\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = (int(b[0][0], 16) << 4)
t += 0x0A00
opcode = f"{t:04x}"
c = re.findall(r'\bJNZA\s*([A-Za-z]\w*)\s*($|;)',line)
if 0 == len(b) and 0 < len(c):
refs.append(c[0][0])
opcode = '8A00(XX)'
b = re.findall(r'\bJNZA\s*#([0-9]+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0])
if t < (1 << 16):
opcode = '8A00'+f"{t:04x}"
b = re.findall(r'\bJNZA\s*#0x([0-9A-Fa-f]+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16)
if t < (1 << 16):
opcode = '8A00'+f"{t:04x}"
if re.search(r'\bJCR\b', line):
b = re.findall(r'\bJCR\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = (int(b[0][0], 16) << 4)
t += 0x0B00
opcode = f"{t:04x}"
c = re.findall(r'\bJCR\s*([A-Za-z]\w*)\s*($|;)',line)
if 0 == len(b) and 0 < len(c):
refs.append(c[0][0])
opcode = 'CB()'
b = re.findall(r'\bJCR\s*\+(\d+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0])
if t < (1 << 7):
t += 0xCB00
opcode = f"{t:04x}"
b = re.findall(r'\bJCR\s*-(\d+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0])
if t <= (1 << 7) and 0 < t:
t = 256 - t
t += 0xCB00
opcode = f"{t:04x}"
if re.search(r'\bJCA\b', line):
b = re.findall(r'\bJCA\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = (int(b[0][0], 16) << 4)
t += 0x0C00
opcode = f"{t:04x}"
c = re.findall(r'\bJCA\s*([A-Za-z]\w*)\s*($|;)',line)
if 0 == len(b) and 0 < len(c):
refs.append(c[0][0])
opcode = '8C00(XX)'
b = re.findall(r'\bJCA\s*#([0-9]+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0])
if t < (1 << 16):
opcode = '8C00'+f"{t:04x}"
b = re.findall(r'\bJCA\s*#0x([0-9A-Fa-f]+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16)
if t < (1 << 16):
opcode = '8C00'+f"{t:04x}"
if re.search(r'\bJNCR\b', line):
b = re.findall(r'\bJNCR\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = (int(b[0][0], 16) << 4)
t += 0x0D00
opcode = f"{t:04x}"
c = re.findall(r'\bJNCR\s*([A-Za-z]\w*)\s*($|;)',line)
if 0 == len(b) and 0 < len(c):
refs.append(c[0][0])
opcode = 'CD()'
b = re.findall(r'\bJNCR\s*\+(\d+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0])
if t < (1 << 7):
t += 0xCD00
opcode = f"{t:04x}"
b = re.findall(r'\bJNCR\s*-(\d+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0])
if t <= (1 << 7) and 0 < t:
t = 256 - t
t += 0xCD00
opcode = f"{t:04x}"
if re.search(r'\bJNCA\b', line):
b = re.findall(r'\bJNCA\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = (int(b[0][0], 16) << 4)
t += 0x0E00
opcode = f"{t:04x}"
c = re.findall(r'\bJNCA\s*([A-Za-z]\w*)\s*($|;)',line)
if 0 == len(b) and 0 < len(c):
refs.append(c[0][0])
opcode = '8E00(XX)'
b = re.findall(r'\bJNCA\s*#([0-9]+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0])
if t < (1 << 16):
opcode = '8E00' + f"{t:04x}"
b = re.findall(r'\bJNCA\s*#0x([0-9A-Fa-f]+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16)
if t < (1 << 16):
opcode = '8E00' + f"{t:04x}"
if re.search(r'\bJR\b', line):
b = re.findall(r'\bJR\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = (int(b[0][0], 16) << 4)
t += 0x0F00
opcode = f"{t:04x}"
c = re.findall(r'\bJR\s*([A-Za-z]\w*)\s*($|;)',line)
if 0 == len(b) and 0 < len(c):
refs.append(c[0][0])
opcode = 'CF()'
b = re.findall(r'\bJR\s*\+(\d+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0])
if t < (1 << 7):
t += 0xCF00
opcode = f"{t:04x}"
b = re.findall(r'\bJR\s*-(\d+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0])
if t <= (1 << 7) and 0 < t:
t = 256 - t
t += 0xCF00
opcode = f"{t:04x}"
if re.search(r'\bJA\b', line):
b = re.findall(r'\bJA\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = (int(b[0][0], 16) << 4)
t += 0x1000
opcode = f"{t:04x}"
c = re.findall(r'\bJA\s*([A-Za-z]\w*)\s*($|;)',line)
if 0 == len(b) and 0 < len(c):
refs.append(c[0][0])
opcode = '9000(XX)'
b = re.findall(r'\bJA\s*#([0-9]+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0])
if t < (1 << 16):
opcode = '9000' + f"{t:04x}"
b = re.findall(r'\bJA\s*#0x([0-9A-Fa-f]+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16)
if t < (1 << 16):
opcode = '9000' + f"{t:04x}"
if re.search(r'\bCR\b', line):
b = re.findall(r'\bCR\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = (int(b[0][0], 16) << 4)
t += 0x1100
opcode = f"{t:04x}"
c = re.findall(r'\bCR\s*([A-Za-z]\w*)\s*($|;)',line)
if 0 == len(b) and 0 < len(c):
refs.append(c[0][0])
opcode = 'D1()'
b = re.findall(r'\bCR\s*\+(\d+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0])
if t < (1 << 7):
t += 0xD100
opcode = f"{t:04x}"
b = re.findall(r'\bCR\s*-(\d+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0])
if t <= (1 << 7) and 0 < t:
t = 256 - t
t += 0xD100
opcode = f"{t:04x}"
if re.search(r'\bCA\b', line):
b = re.findall(r'\bCA\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = (int(b[0][0], 16) << 4)
t += 0x1200
opcode = f"{t:04x}"
c = re.findall(r'\bCA\s*([A-Za-z]\w*)\s*($|;)',line)
if 0 == len(b) and 0 < len(c):
refs.append(c[0][0])
opcode = '9200(XX)'
b = re.findall(r'\bCA\s*#([0-9]+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0])
if t < (1 << 16):
opcode = '9200' + f"{t:04x}"
b = re.findall(r'\bCA\s*#0x([0-9A-Fa-f]+)\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16)
if t < (1 << 16):
opcode = '9200' + f"{t:04x}"
if re.search(r'\bRET\b\s*($|;)', line):
opcode = '1300'
if re.search(r'\bSTP\b\s*($|;)', line):
opcode = '1400'
if re.search(r'\bFP\b', line):
b = re.findall(r'\bFP\W*R([0-9A-Fa-f]),\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16) + (int(b[0][1], 16) << 4)
t += 24<<8
opcode = f"{t:04x}"
if re.search(r'\bFPRR\b', line):
b = re.findall(r'\bFPRR\W*R([0-7]),\W*R([0-7]),\W*R([0-7])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16) + (int(b[0][1], 16) << 3) + (int(b[0][2], 16) << 6)
t += 0x4000 | (24<<8)
opcode = f"{t:04x}"
if re.search(r'\bMM1\b', line):
b = re.findall(r'\bMM1\W*R([0-9A-Fa-f]),\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16) + (int(b[0][1], 16) << 4)
t += 26<<8
opcode = f"{t:04x}"
if re.search(r'\bMM\b', line):
b = re.findall(r'\bMM\W*R([0-7]),\W*R([0-7]),\W*R([0-7])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16) + (int(b[0][1], 16) << 3) + (int(b[0][2], 16) << 6)
t += 0x4000 | (26<<8)
opcode = f"{t:04x}"
if re.search(r'\bEDIV\b', line):
b = re.findall(r'\bEDIV\W*R([0-7]),\W*R([0-7]),\W*R([0-7])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16) + (int(b[0][1], 16) << 3) + (int(b[0][2], 16) << 6)
t += 0x4000 | (28<<8)
opcode = f"{t:04x}"
if re.search(r'\bMPOW\b', line):
b = re.findall(r'\bMPOW\W*R([0-9A-Fa-f]),\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16) + (int(b[0][1], 16) << 4)
t += 25<<8
opcode = f"{t:04x}"
if re.search(r'\bMOVRR\b', line):
b = re.findall(r'\bMOVRR\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16)
t += 28<<8
opcode = f"{t:04x}"
if re.search(r'\bMR\b', line):
b = re.findall(r'\bMR\W*R([0-9A-Fa-f])\s*($|;)',line)
if 0 < len(b):
t = int(b[0][0], 16)
t += 27<<8
opcode = f"{t:04x}"
if 'FFFF' == opcode:
print(f'invalid instruction {line}')
res += opcode
# Handle labels
b = re.split(r"\(",res)
res = b[0]
for i in range(1, len(b)):
s = len(res)
PC = s >> 2
b2 = re.split(r"\)",b[i])
label = refs[i-1]
if label in labels:
t = labels[label]
# is jump relative?
if (s >> 1) & 1:
PC += 1
t = t - PC
if t < 128 and t >= -128:
if t < 0:
t += (1 << 8)
res += f"{t:02X}"
else:
print(f"label {label} out of reach")
else:
if t < (1<<16):
res += f"{t:04x}"
else:
print(f"label {label} out of reach")
else:
print(f"missing label {label}")
res += b2[1]
return res
if __name__ == "__main__":
code = open(sys.argv[1], "r").read().splitlines()
code = assembly(code)
print(code)
crypto_accelerator.py
import random
class Fp_machine:
def __init__(self, p, minWorkingSize, RR=0, logWordSize=6):
self.logWordSize = logWordSize
self.wordSize = 1<<logWordSize
self.workingSize = ((minWorkingSize+3+((1<<logWordSize)-1))>>logWordSize)<<logWordSize
self.module = p
self.v = NegInvertModuloPower2(p,self.wordSize)
if RR:
self.RR = RR
else:
self.RR = self.computeRR()
self.R = self.MM(self.RR,1)
def display(self):
print(f"word size: {self.wordSize}")
print(f"workingSize:{self.workingSize}")
print(f"module: {hex(self.module)}")
print(f"RR: {hex(self.RR)}")
print(f"v: {hex(self.v)}")
def check(self):
if (not self.v) or self.v == 0:
print("error v")
return False
if (not self.module) or self.module == 0:
print("error module")
return False
if (self.module&1) == 0:
print("module not odd")
return False
return True
#Montgomery Multiplication : a*b/R mod module
def MM(self, a, b):
if b==1:
return self.multByOne(a)
if not self.check():
return False
res = 0
wordMask = (1<<self.wordSize)-1
for _ in range(self.workingSize>>self.logWordSize):
currentWord = b&wordMask
b >>= self.wordSize
res += currentWord*a
u = ((res&wordMask)*self.v)&wordMask
res += u*self.module
res >>= self.wordSize
return res
def multByOne(self,a):
if not self.check():
return False
res = a
wordMask = (1<<self.wordSize)-1
for _ in range(self.workingSize>>self.logWordSize):
u = ((res&wordMask)*self.v)&wordMask
res += u*self.module
res >>= self.wordSize
return res
#Compute R*R mod module
def computeRR(self):
if not self.check():
return False
wordCount = 0
lastWord = 0
tmp = self.module
while tmp > 0:
lastWord = tmp
tmp >>= self.wordSize
wordCount += 1
clz = CountLeadingZeroes(lastWord,self.wordSize)
R = (1<<self.workingSize)-1
R ^= self.module
R ^= 1
RR = R
align = self.module<<(clz+self.workingSize-(wordCount<<self.logWordSize))
tradeoff = 5
if self.logWordSize < tradeoff:
tradeoff = self.logWordSize
shift = self.workingSize>>tradeoff
if RR > align:
RR -= align
for _ in range(shift):
RR += RR
if RR > align:
RR -= align
for _ in range(tradeoff):
RR = self.MM(RR,RR)
RR = self.MM(RR,RR)
RR = self.MM(RR,1)
return RR
def MPow(self,m,e):
if (1==e):
return m
res = self.R
a = m
while e > 0:
if (e&1==1):
res = self.MM(res,a)
a = self.MM(a,a)
e >>= 1
return res
def RemoveTrailingZeroes(a):
ctz = 0
while a&1==0:
a>>=1
ctz+=1
return ctz,a
#count leading zeroes for one word, according to the bitsize of a word
def CountLeadingZeroes(a,wordsize):
if a==0:
return wordsize
a &= (1<<wordsize)-1
res = 0
for i in range(wordsize-1,-1,-1):
if a&(1<<i) != 0:
return res
res += 1
return res
# return -1/a mod 2**n
def NegInvertModuloPower2(a,n):
if a&1==0:
return False
a &=((1<<n)-1)
res = 1
i = 1
while i<n :
res = res*(2+a*res)
i<<=1
return res&((1<<n)-1)
def InvertModuloPower2(a,n):
return NegInvertModuloPower2((1<<n)-a,n)
def ExactDivision(a,b,bitsize=32):
while a&1 == b&1 and a&1==0:
a >>= 1
b >>= 1
res = 0
k = 0
i = InvertModuloPower2(b,bitsize)
while a > 0:
r = (i*a)&((1<<bitsize)-1)
res += r<<(k*bitsize)
a -= r*b
a >>= bitsize
k+=1
return res
def MillerRabin_round(a,machine):
p = machine.module
ctz, e = RemoveTrailingZeroes(p-1)
a = machine.MPow(a,e)
t = machine.MM(a,1)
if (1 == t) or (p-1 == t):
return True
for _ in range(ctz-1):
y = machine.MM(a,a)
t = machine.MM(y,1)
if (1 == t):
return False
if (p-1 == t):
return True
a = y
if not (p-1 == t):
return False
return True
def MillerRabin(p):
if p < 2:
return False
if (p&1)==0:
return False
if p < 9:
return True
machine = Fp_machine(p, p.bit_length())
if not MillerRabin_round(2,machine):
return False
nb_test = 49
if p.bit_length() >= 100:
nb_test = 37
if p.bit_length() >= 140:
nb_test = 31
if p.bit_length() >= 170:
nb_test = 26
if p.bit_length() >= 1024:
nb_test = 3
if p.bit_length() >= 2048:
nb_test = 2
for _ in range(nb_test):
a = random.randrange(3,p-1)
if not MillerRabin_round(a,machine):
return False
return True
machine_faulted.py
from machine import Machine
from random import randrange
class FaultedMachine(Machine):
def __init__(self, code, list_faults = []):
super().__init__(code)
self.list_faults = list_faults
def finalize_move(self):
if self.nbInstruction in self.list_faults:
self.a = randrange(1 << self.module.bit_length())
super().finalize_move()
mille-fautes.py
# Python regular imports
import gmpy2
from random import randrange
from Crypto.Util.number import getPrime
# Public files (challenge specific)
from machine import Machine
from machine_faulted import FaultedMachine
from assembly import assembly
def RSAKeyGen(nbits, e):
N = 2
while N.bit_length() != nbits:
p = getPrime(nbits // 2)
q = getPrime(nbits // 2)
N = p * q
d = gmpy2.invert(e, (p - 1) * (q - 1))
return N, d
def authenticate(c, M, N, d):
c.R5 = d
c.R6 = M
c.exponent = 2
c.module = N
c.runCode()
if c.error:
return 0, c.nbInstruction
return c.R2, c.nbInstruction
def verifyAuthentication(s, M, N, e):
return M == gmpy2.powmod(s, e, N)
if __name__ == "__main__":
nbits = 1024
e = 65537
N, d = RSAKeyGen(nbits, e)
print(f"{N = }")
code = open("RSA.asm").read().splitlines()
code = assembly(code)
for i in range(nbits):
print("Enter the index of the instruction you want to fault")
fault = [ int(input(">>> ")) ]
machine = FaultedMachine(code, fault)
M = randrange(N)
s, n = authenticate(machine, M, N, d)
print(f"{i:5d} {fault[0]:5d} {n:5d} {verifyAuthentication(s, M, N, e)}")
mille-fautes.txt
N = 138382400518283433090436751179684661427490347924193727098510273599025233276466314824189344030612472818210363322534176328466290613590292789057447894776397515098797067477327506046346083228776836819971005224100849669209983298891971162523901798545349971090413277577634995635066092475983206870690345198501384096463
Enter the index of the instruction you want to fault
>>> 0 3 11268 False
Enter the index of the instruction you want to fault
>>> 1 14 11268 True
Enter the index of the instruction you want to fault
>>> 2 25 11268 True
Enter the index of the instruction you want to fault
>>> 3 36 11268 True
Enter the index of the instruction you want to fault
>>> 4 47 11268 False
Enter the index of the instruction you want to fault
>>> 5 58 11268 True
Enter the index of the instruction you want to fault
>>> 6 69 11268 False
Enter the index of the instruction you want to fault
>>> 7 80 11268 False
Enter the index of the instruction you want to fault
>>> 8 91 11268 False
Enter the index of the instruction you want to fault
>>> 9 102 11268 False
Enter the index of the instruction you want to fault
>>> 10 113 11268 False
Enter the index of the instruction you want to fault
>>> 11 124 11268 True
Enter the index of the instruction you want to fault
>>> 12 135 11268 True
Enter the index of the instruction you want to fault
>>> 13 146 11268 True
Enter the index of the instruction you want to fault
>>> 14 157 11268 True
Enter the index of the instruction you want to fault
>>> 15 168 11268 False
Enter the index of the instruction you want to fault
>>> 16 179 11268 False
Enter the index of the instruction you want to fault
>>> 17 190 11268 True
Enter the index of the instruction you want to fault
>>> 18 201 11268 False
Enter the index of the instruction you want to fault
>>> 19 212 11268 False
Enter the index of the instruction you want to fault
>>> 20 223 11268 False
Enter the index of the instruction you want to fault
>>> 21 234 11268 True
Enter the index of the instruction you want to fault
>>> 22 245 11268 True
Enter the index of the instruction you want to fault
>>> 23 256 11268 True
Enter the index of the instruction you want to fault
>>> 24 267 11268 False
Enter the index of the instruction you want to fault
>>> 25 278 11268 True
Enter the index of the instruction you want to fault
>>> 26 289 11268 False
Enter the index of the instruction you want to fault
>>> 27 300 11268 False
Enter the index of the instruction you want to fault
>>> 28 311 11268 False
Enter the index of the instruction you want to fault
>>> 29 322 11268 False
Enter the index of the instruction you want to fault
>>> 30 333 11268 False
Enter the index of the instruction you want to fault
>>> 31 344 11268 True
Enter the index of the instruction you want to fault
>>> 32 355 11268 False
Enter the index of the instruction you want to fault
>>> 33 366 11268 True
Enter the index of the instruction you want to fault
>>> 34 377 11268 True
Enter the index of the instruction you want to fault
>>> 35 388 11268 True
Enter the index of the instruction you want to fault
>>> 36 399 11268 True
Enter the index of the instruction you want to fault
>>> 37 410 11268 True
Enter the index of the instruction you want to fault
>>> 38 421 11268 True
Enter the index of the instruction you want to fault
>>> 39 432 11268 False
Enter the index of the instruction you want to fault
>>> 40 443 11268 True
Enter the index of the instruction you want to fault
>>> 41 454 11268 True
Enter the index of the instruction you want to fault
>>> 42 465 11268 True
Enter the index of the instruction you want to fault
>>> 43 476 11268 True
Enter the index of the instruction you want to fault
>>> 44 487 11268 True
Enter the index of the instruction you want to fault
>>> 45 498 11268 False
Enter the index of the instruction you want to fault
>>> 46 509 11268 False
Enter the index of the instruction you want to fault
>>> 47 520 11268 True
Enter the index of the instruction you want to fault
>>> 48 531 11268 True
Enter the index of the instruction you want to fault
>>> 49 542 11268 True
Enter the index of the instruction you want to fault
>>> 50 553 11268 True
Enter the index of the instruction you want to fault
>>> 51 564 11268 True
Enter the index of the instruction you want to fault
>>> 52 575 11268 False
Enter the index of the instruction you want to fault
>>> 53 586 11268 False
Enter the index of the instruction you want to fault
>>> 54 597 11268 True
Enter the index of the instruction you want to fault
>>> 55 608 11268 True
Enter the index of the instruction you want to fault
>>> 56 619 11268 True
Enter the index of the instruction you want to fault
>>> 57 630 11268 False
Enter the index of the instruction you want to fault
>>> 58 641 11268 True
Enter the index of the instruction you want to fault
>>> 59 652 11268 False
Enter the index of the instruction you want to fault
>>> 60 663 11268 False
Enter the index of the instruction you want to fault
>>> 61 674 11268 True
Enter the index of the instruction you want to fault
>>> 62 685 11268 False
Enter the index of the instruction you want to fault
>>> 63 696 11268 False
Enter the index of the instruction you want to fault
>>> 64 707 11268 True
Enter the index of the instruction you want to fault
>>> 65 718 11268 False
Enter the index of the instruction you want to fault
>>> 66 729 11268 True
Enter the index of the instruction you want to fault
>>> 67 740 11268 False
Enter the index of the instruction you want to fault
>>> 68 751 11268 False
Enter the index of the instruction you want to fault
>>> 69 762 11268 True
Enter the index of the instruction you want to fault
>>> 70 773 11268 True
Enter the index of the instruction you want to fault
>>> 71 784 11268 False
Enter the index of the instruction you want to fault
>>> 72 795 11268 False
Enter the index of the instruction you want to fault
>>> 73 806 11268 False
Enter the index of the instruction you want to fault
>>> 74 817 11268 True
Enter the index of the instruction you want to fault
>>> 75 828 11268 True
Enter the index of the instruction you want to fault
>>> 76 839 11268 True
Enter the index of the instruction you want to fault
>>> 77 850 11268 False
Enter the index of the instruction you want to fault
>>> 78 861 11268 False
Enter the index of the instruction you want to fault
>>> 79 872 11268 False
Enter the index of the instruction you want to fault
>>> 80 883 11268 True
Enter the index of the instruction you want to fault
>>> 81 894 11268 False
Enter the index of the instruction you want to fault
>>> 82 905 11268 False
Enter the index of the instruction you want to fault
>>> 83 916 11268 True
Enter the index of the instruction you want to fault
>>> 84 927 11268 True
Enter the index of the instruction you want to fault
>>> 85 938 11268 True
Enter the index of the instruction you want to fault
>>> 86 949 11268 False
Enter the index of the instruction you want to fault
>>> 87 960 11268 False
Enter the index of the instruction you want to fault
>>> 88 971 11268 True
Enter the index of the instruction you want to fault
>>> 89 982 11268 True
Enter the index of the instruction you want to fault
>>> 90 993 11268 False
Enter the index of the instruction you want to fault
>>> 91 1004 11268 True
Enter the index of the instruction you want to fault
>>> 92 1015 11268 False
Enter the index of the instruction you want to fault
>>> 93 1026 11268 False
Enter the index of the instruction you want to fault
>>> 94 1037 11268 False
Enter the index of the instruction you want to fault
>>> 95 1048 11268 False
Enter the index of the instruction you want to fault
>>> 96 1059 11268 False
Enter the index of the instruction you want to fault
>>> 97 1070 11268 False
Enter the index of the instruction you want to fault
>>> 98 1081 11268 False
Enter the index of the instruction you want to fault
>>> 99 1092 11268 True
Enter the index of the instruction you want to fault
>>> 100 1103 11268 False
Enter the index of the instruction you want to fault
>>> 101 1114 11268 True
Enter the index of the instruction you want to fault
>>> 102 1125 11268 True
Enter the index of the instruction you want to fault
>>> 103 1136 11268 False
Enter the index of the instruction you want to fault
>>> 104 1147 11268 True
Enter the index of the instruction you want to fault
>>> 105 1158 11268 False
Enter the index of the instruction you want to fault
>>> 106 1169 11268 False
Enter the index of the instruction you want to fault
>>> 107 1180 11268 False
Enter the index of the instruction you want to fault
>>> 108 1191 11268 False
Enter the index of the instruction you want to fault
>>> 109 1202 11268 True
Enter the index of the instruction you want to fault
>>> 110 1213 11268 True
Enter the index of the instruction you want to fault
>>> 111 1224 11268 False
Enter the index of the instruction you want to fault
>>> 112 1235 11268 True
Enter the index of the instruction you want to fault
>>> 113 1246 11268 False
Enter the index of the instruction you want to fault
>>> 114 1257 11268 True
Enter the index of the instruction you want to fault
>>> 115 1268 11268 False
Enter the index of the instruction you want to fault
>>> 116 1279 11268 False
Enter the index of the instruction you want to fault
>>> 117 1290 11268 True
Enter the index of the instruction you want to fault
>>> 118 1301 11268 True
Enter the index of the instruction you want to fault
>>> 119 1312 11268 True
Enter the index of the instruction you want to fault
>>> 120 1323 11268 False
Enter the index of the instruction you want to fault
>>> 121 1334 11268 False
Enter the index of the instruction you want to fault
>>> 122 1345 11268 True
Enter the index of the instruction you want to fault
>>> 123 1356 11268 False
Enter the index of the instruction you want to fault
>>> 124 1367 11268 True
Enter the index of the instruction you want to fault
>>> 125 1378 11268 True
Enter the index of the instruction you want to fault
>>> 126 1389 11268 True
Enter the index of the instruction you want to fault
>>> 127 1400 11268 True
Enter the index of the instruction you want to fault
>>> 128 1411 11268 True
Enter the index of the instruction you want to fault
>>> 129 1422 11268 True
Enter the index of the instruction you want to fault
>>> 130 1433 11268 False
Enter the index of the instruction you want to fault
>>> 131 1444 11268 True
Enter the index of the instruction you want to fault
>>> 132 1455 11268 False
Enter the index of the instruction you want to fault
>>> 133 1466 11268 False
Enter the index of the instruction you want to fault
>>> 134 1477 11268 False
Enter the index of the instruction you want to fault
>>> 135 1488 11268 False
Enter the index of the instruction you want to fault
>>> 136 1499 11268 True
Enter the index of the instruction you want to fault
>>> 137 1510 11268 True
Enter the index of the instruction you want to fault
>>> 138 1521 11268 True
Enter the index of the instruction you want to fault
>>> 139 1532 11268 False
Enter the index of the instruction you want to fault
>>> 140 1543 11268 False
Enter the index of the instruction you want to fault
>>> 141 1554 11268 False
Enter the index of the instruction you want to fault
>>> 142 1565 11268 False
Enter the index of the instruction you want to fault
>>> 143 1576 11268 True
Enter the index of the instruction you want to fault
>>> 144 1587 11268 True
Enter the index of the instruction you want to fault
>>> 145 1598 11268 False
Enter the index of the instruction you want to fault
>>> 146 1609 11268 False
Enter the index of the instruction you want to fault
>>> 147 1620 11268 True
Enter the index of the instruction you want to fault
>>> 148 1631 11268 False
Enter the index of the instruction you want to fault
>>> 149 1642 11268 False
Enter the index of the instruction you want to fault
>>> 150 1653 11268 True
Enter the index of the instruction you want to fault
>>> 151 1664 11268 False
Enter the index of the instruction you want to fault
>>> 152 1675 11268 False
Enter the index of the instruction you want to fault
>>> 153 1686 11268 False
Enter the index of the instruction you want to fault
>>> 154 1697 11268 True
Enter the index of the instruction you want to fault
>>> 155 1708 11268 False
Enter the index of the instruction you want to fault
>>> 156 1719 11268 True
Enter the index of the instruction you want to fault
>>> 157 1730 11268 False
Enter the index of the instruction you want to fault
>>> 158 1741 11268 True
Enter the index of the instruction you want to fault
>>> 159 1752 11268 True
Enter the index of the instruction you want to fault
>>> 160 1763 11268 True
Enter the index of the instruction you want to fault
>>> 161 1774 11268 False
Enter the index of the instruction you want to fault
>>> 162 1785 11268 True
Enter the index of the instruction you want to fault
>>> 163 1796 11268 True
Enter the index of the instruction you want to fault
>>> 164 1807 11268 True
Enter the index of the instruction you want to fault
>>> 165 1818 11268 False
Enter the index of the instruction you want to fault
>>> 166 1829 11268 False
Enter the index of the instruction you want to fault
>>> 167 1840 11268 False
Enter the index of the instruction you want to fault
>>> 168 1851 11268 True
Enter the index of the instruction you want to fault
>>> 169 1862 11268 False
Enter the index of the instruction you want to fault
>>> 170 1873 11268 False
Enter the index of the instruction you want to fault
>>> 171 1884 11268 False
Enter the index of the instruction you want to fault
>>> 172 1895 11268 False
Enter the index of the instruction you want to fault
>>> 173 1906 11268 True
Enter the index of the instruction you want to fault
>>> 174 1917 11268 False
Enter the index of the instruction you want to fault
>>> 175 1928 11268 False
Enter the index of the instruction you want to fault
>>> 176 1939 11268 False
Enter the index of the instruction you want to fault
>>> 177 1950 11268 False
Enter the index of the instruction you want to fault
>>> 178 1961 11268 False
Enter the index of the instruction you want to fault
>>> 179 1972 11268 True
Enter the index of the instruction you want to fault
>>> 180 1983 11268 True
Enter the index of the instruction you want to fault
>>> 181 1994 11268 False
Enter the index of the instruction you want to fault
>>> 182 2005 11268 True
Enter the index of the instruction you want to fault
>>> 183 2016 11268 False
Enter the index of the instruction you want to fault
>>> 184 2027 11268 False
Enter the index of the instruction you want to fault
>>> 185 2038 11268 False
Enter the index of the instruction you want to fault
>>> 186 2049 11268 False
Enter the index of the instruction you want to fault
>>> 187 2060 11268 True
Enter the index of the instruction you want to fault
>>> 188 2071 11268 False
Enter the index of the instruction you want to fault
>>> 189 2082 11268 True
Enter the index of the instruction you want to fault
>>> 190 2093 11268 True
Enter the index of the instruction you want to fault
>>> 191 2104 11268 True
Enter the index of the instruction you want to fault
>>> 192 2115 11268 False
Enter the index of the instruction you want to fault
>>> 193 2126 11268 False
Enter the index of the instruction you want to fault
>>> 194 2137 11268 True
Enter the index of the instruction you want to fault
>>> 195 2148 11268 True
Enter the index of the instruction you want to fault
>>> 196 2159 11268 True
Enter the index of the instruction you want to fault
>>> 197 2170 11268 False
Enter the index of the instruction you want to fault
>>> 198 2181 11268 True
Enter the index of the instruction you want to fault
>>> 199 2192 11268 False
Enter the index of the instruction you want to fault
>>> 200 2203 11268 False
Enter the index of the instruction you want to fault
>>> 201 2214 11268 True
Enter the index of the instruction you want to fault
>>> 202 2225 11268 False
Enter the index of the instruction you want to fault
>>> 203 2236 11268 False
Enter the index of the instruction you want to fault
>>> 204 2247 11268 False
Enter the index of the instruction you want to fault
>>> 205 2258 11268 True
Enter the index of the instruction you want to fault
>>> 206 2269 11268 True
Enter the index of the instruction you want to fault
>>> 207 2280 11268 False
Enter the index of the instruction you want to fault
>>> 208 2291 11268 False
Enter the index of the instruction you want to fault
>>> 209 2302 11268 False
Enter the index of the instruction you want to fault
>>> 210 2313 11268 True
Enter the index of the instruction you want to fault
>>> 211 2324 11268 True
Enter the index of the instruction you want to fault
>>> 212 2335 11268 False
Enter the index of the instruction you want to fault
>>> 213 2346 11268 True
Enter the index of the instruction you want to fault
>>> 214 2357 11268 False
Enter the index of the instruction you want to fault
>>> 215 2368 11268 True
Enter the index of the instruction you want to fault
>>> 216 2379 11268 False
Enter the index of the instruction you want to fault
>>> 217 2390 11268 False
Enter the index of the instruction you want to fault
>>> 218 2401 11268 True
Enter the index of the instruction you want to fault
>>> 219 2412 11268 True
Enter the index of the instruction you want to fault
>>> 220 2423 11268 False
Enter the index of the instruction you want to fault
>>> 221 2434 11268 True
Enter the index of the instruction you want to fault
>>> 222 2445 11268 False
Enter the index of the instruction you want to fault
>>> 223 2456 11268 True
Enter the index of the instruction you want to fault
>>> 224 2467 11268 False
Enter the index of the instruction you want to fault
>>> 225 2478 11268 False
Enter the index of the instruction you want to fault
>>> 226 2489 11268 False
Enter the index of the instruction you want to fault
>>> 227 2500 11268 False
Enter the index of the instruction you want to fault
>>> 228 2511 11268 True
Enter the index of the instruction you want to fault
>>> 229 2522 11268 False
Enter the index of the instruction you want to fault
>>> 230 2533 11268 True
Enter the index of the instruction you want to fault
>>> 231 2544 11268 True
Enter the index of the instruction you want to fault
>>> 232 2555 11268 False
Enter the index of the instruction you want to fault
>>> 233 2566 11268 False
Enter the index of the instruction you want to fault
>>> 234 2577 11268 True
Enter the index of the instruction you want to fault
>>> 235 2588 11268 True
Enter the index of the instruction you want to fault
>>> 236 2599 11268 True
Enter the index of the instruction you want to fault
>>> 237 2610 11268 True
Enter the index of the instruction you want to fault
>>> 238 2621 11268 True
Enter the index of the instruction you want to fault
>>> 239 2632 11268 True
Enter the index of the instruction you want to fault
>>> 240 2643 11268 True
Enter the index of the instruction you want to fault
>>> 241 2654 11268 False
Enter the index of the instruction you want to fault
>>> 242 2665 11268 False
Enter the index of the instruction you want to fault
>>> 243 2676 11268 True
Enter the index of the instruction you want to fault
>>> 244 2687 11268 True
Enter the index of the instruction you want to fault
>>> 245 2698 11268 False
Enter the index of the instruction you want to fault
>>> 246 2709 11268 True
Enter the index of the instruction you want to fault
>>> 247 2720 11268 True
Enter the index of the instruction you want to fault
>>> 248 2731 11268 True
Enter the index of the instruction you want to fault
>>> 249 2742 11268 True
Enter the index of the instruction you want to fault
>>> 250 2753 11268 True
Enter the index of the instruction you want to fault
>>> 251 2764 11268 False
Enter the index of the instruction you want to fault
>>> 252 2775 11268 True
Enter the index of the instruction you want to fault
>>> 253 2786 11268 False
Enter the index of the instruction you want to fault
>>> 254 2797 11268 True
Enter the index of the instruction you want to fault
>>> 255 2808 11268 False
Enter the index of the instruction you want to fault
>>> 256 2819 11268 False
Enter the index of the instruction you want to fault
>>> 257 2830 11268 False
Enter the index of the instruction you want to fault
>>> 258 2841 11268 True
Enter the index of the instruction you want to fault
>>> 259 2852 11268 False
Enter the index of the instruction you want to fault
>>> 260 2863 11268 True
Enter the index of the instruction you want to fault
>>> 261 2874 11268 True
Enter the index of the instruction you want to fault
>>> 262 2885 11268 True
Enter the index of the instruction you want to fault
>>> 263 2896 11268 False
Enter the index of the instruction you want to fault
>>> 264 2907 11268 False
Enter the index of the instruction you want to fault
>>> 265 2918 11268 False
Enter the index of the instruction you want to fault
>>> 266 2929 11268 True
Enter the index of the instruction you want to fault
>>> 267 2940 11268 True
Enter the index of the instruction you want to fault
>>> 268 2951 11268 False
Enter the index of the instruction you want to fault
>>> 269 2962 11268 False
Enter the index of the instruction you want to fault
>>> 270 2973 11268 False
Enter the index of the instruction you want to fault
>>> 271 2984 11268 True
Enter the index of the instruction you want to fault
>>> 272 2995 11268 False
Enter the index of the instruction you want to fault
>>> 273 3006 11268 False
Enter the index of the instruction you want to fault
>>> 274 3017 11268 False
Enter the index of the instruction you want to fault
>>> 275 3028 11268 False
Enter the index of the instruction you want to fault
>>> 276 3039 11268 False
Enter the index of the instruction you want to fault
>>> 277 3050 11268 False
Enter the index of the instruction you want to fault
>>> 278 3061 11268 False
Enter the index of the instruction you want to fault
>>> 279 3072 11268 True
Enter the index of the instruction you want to fault
>>> 280 3083 11268 False
Enter the index of the instruction you want to fault
>>> 281 3094 11268 True
Enter the index of the instruction you want to fault
>>> 282 3105 11268 False
Enter the index of the instruction you want to fault
>>> 283 3116 11268 True
Enter the index of the instruction you want to fault
>>> 284 3127 11268 True
Enter the index of the instruction you want to fault
>>> 285 3138 11268 True
Enter the index of the instruction you want to fault
>>> 286 3149 11268 False
Enter the index of the instruction you want to fault
>>> 287 3160 11268 True
Enter the index of the instruction you want to fault
>>> 288 3171 11268 False
Enter the index of the instruction you want to fault
>>> 289 3182 11268 True
Enter the index of the instruction you want to fault
>>> 290 3193 11268 False
Enter the index of the instruction you want to fault
>>> 291 3204 11268 False
Enter the index of the instruction you want to fault
>>> 292 3215 11268 True
Enter the index of the instruction you want to fault
>>> 293 3226 11268 False
Enter the index of the instruction you want to fault
>>> 294 3237 11268 False
Enter the index of the instruction you want to fault
>>> 295 3248 11268 False
Enter the index of the instruction you want to fault
>>> 296 3259 11268 True
Enter the index of the instruction you want to fault
>>> 297 3270 11268 True
Enter the index of the instruction you want to fault
>>> 298 3281 11268 True
Enter the index of the instruction you want to fault
>>> 299 3292 11268 False
Enter the index of the instruction you want to fault
>>> 300 3303 11268 True
Enter the index of the instruction you want to fault
>>> 301 3314 11268 True
Enter the index of the instruction you want to fault
>>> 302 3325 11268 True
Enter the index of the instruction you want to fault
>>> 303 3336 11268 True
Enter the index of the instruction you want to fault
>>> 304 3347 11268 False
Enter the index of the instruction you want to fault
>>> 305 3358 11268 False
Enter the index of the instruction you want to fault
>>> 306 3369 11268 True
Enter the index of the instruction you want to fault
>>> 307 3380 11268 True
Enter the index of the instruction you want to fault
>>> 308 3391 11268 True
Enter the index of the instruction you want to fault
>>> 309 3402 11268 False
Enter the index of the instruction you want to fault
>>> 310 3413 11268 True
Enter the index of the instruction you want to fault
>>> 311 3424 11268 True
Enter the index of the instruction you want to fault
>>> 312 3435 11268 True
Enter the index of the instruction you want to fault
>>> 313 3446 11268 False
Enter the index of the instruction you want to fault
>>> 314 3457 11268 False
Enter the index of the instruction you want to fault
>>> 315 3468 11268 True
Enter the index of the instruction you want to fault
>>> 316 3479 11268 True
Enter the index of the instruction you want to fault
>>> 317 3490 11268 False
Enter the index of the instruction you want to fault
>>> 318 3501 11268 True
Enter the index of the instruction you want to fault
>>> 319 3512 11268 True
Enter the index of the instruction you want to fault
>>> 320 3523 11268 True
Enter the index of the instruction you want to fault
>>> 321 3534 11268 False
Enter the index of the instruction you want to fault
>>> 322 3545 11268 False
Enter the index of the instruction you want to fault
>>> 323 3556 11268 False
Enter the index of the instruction you want to fault
>>> 324 3567 11268 False
Enter the index of the instruction you want to fault
>>> 325 3578 11268 False
Enter the index of the instruction you want to fault
>>> 326 3589 11268 False
Enter the index of the instruction you want to fault
>>> 327 3600 11268 True
Enter the index of the instruction you want to fault
>>> 328 3611 11268 True
Enter the index of the instruction you want to fault
>>> 329 3622 11268 True
Enter the index of the instruction you want to fault
>>> 330 3633 11268 True
Enter the index of the instruction you want to fault
>>> 331 3644 11268 True
Enter the index of the instruction you want to fault
>>> 332 3655 11268 False
Enter the index of the instruction you want to fault
>>> 333 3666 11268 True
Enter the index of the instruction you want to fault
>>> 334 3677 11268 False
Enter the index of the instruction you want to fault
>>> 335 3688 11268 False
Enter the index of the instruction you want to fault
>>> 336 3699 11268 False
Enter the index of the instruction you want to fault
>>> 337 3710 11268 False
Enter the index of the instruction you want to fault
>>> 338 3721 11268 False
Enter the index of the instruction you want to fault
>>> 339 3732 11268 False
Enter the index of the instruction you want to fault
>>> 340 3743 11268 True
Enter the index of the instruction you want to fault
>>> 341 3754 11268 True
Enter the index of the instruction you want to fault
>>> 342 3765 11268 True
Enter the index of the instruction you want to fault
>>> 343 3776 11268 False
Enter the index of the instruction you want to fault
>>> 344 3787 11268 True
Enter the index of the instruction you want to fault
>>> 345 3798 11268 False
Enter the index of the instruction you want to fault
>>> 346 3809 11268 False
Enter the index of the instruction you want to fault
>>> 347 3820 11268 True
Enter the index of the instruction you want to fault
>>> 348 3831 11268 True
Enter the index of the instruction you want to fault
>>> 349 3842 11268 True
Enter the index of the instruction you want to fault
>>> 350 3853 11268 False
Enter the index of the instruction you want to fault
>>> 351 3864 11268 False
Enter the index of the instruction you want to fault
>>> 352 3875 11268 True
Enter the index of the instruction you want to fault
>>> 353 3886 11268 True
Enter the index of the instruction you want to fault
>>> 354 3897 11268 True
Enter the index of the instruction you want to fault
>>> 355 3908 11268 False
Enter the index of the instruction you want to fault
>>> 356 3919 11268 True
Enter the index of the instruction you want to fault
>>> 357 3930 11268 True
Enter the index of the instruction you want to fault
>>> 358 3941 11268 False
Enter the index of the instruction you want to fault
>>> 359 3952 11268 True
Enter the index of the instruction you want to fault
>>> 360 3963 11268 True
Enter the index of the instruction you want to fault
>>> 361 3974 11268 False
Enter the index of the instruction you want to fault
>>> 362 3985 11268 True
Enter the index of the instruction you want to fault
>>> 363 3996 11268 True
Enter the index of the instruction you want to fault
>>> 364 4007 11268 False
Enter the index of the instruction you want to fault
>>> 365 4018 11268 False
Enter the index of the instruction you want to fault
>>> 366 4029 11268 False
Enter the index of the instruction you want to fault
>>> 367 4040 11268 True
Enter the index of the instruction you want to fault
>>> 368 4051 11268 False
Enter the index of the instruction you want to fault
>>> 369 4062 11268 False
Enter the index of the instruction you want to fault
>>> 370 4073 11268 False
Enter the index of the instruction you want to fault
>>> 371 4084 11268 True
Enter the index of the instruction you want to fault
>>> 372 4095 11268 False
Enter the index of the instruction you want to fault
>>> 373 4106 11268 False
Enter the index of the instruction you want to fault
>>> 374 4117 11268 True
Enter the index of the instruction you want to fault
>>> 375 4128 11268 True
Enter the index of the instruction you want to fault
>>> 376 4139 11268 True
Enter the index of the instruction you want to fault
>>> 377 4150 11268 False
Enter the index of the instruction you want to fault
>>> 378 4161 11268 True
Enter the index of the instruction you want to fault
>>> 379 4172 11268 True
Enter the index of the instruction you want to fault
>>> 380 4183 11268 False
Enter the index of the instruction you want to fault
>>> 381 4194 11268 False
Enter the index of the instruction you want to fault
>>> 382 4205 11268 True
Enter the index of the instruction you want to fault
>>> 383 4216 11268 False
Enter the index of the instruction you want to fault
>>> 384 4227 11268 False
Enter the index of the instruction you want to fault
>>> 385 4238 11268 True
Enter the index of the instruction you want to fault
>>> 386 4249 11268 True
Enter the index of the instruction you want to fault
>>> 387 4260 11268 True
Enter the index of the instruction you want to fault
>>> 388 4271 11268 False
Enter the index of the instruction you want to fault
>>> 389 4282 11268 True
Enter the index of the instruction you want to fault
>>> 390 4293 11268 True
Enter the index of the instruction you want to fault
>>> 391 4304 11268 True
Enter the index of the instruction you want to fault
>>> 392 4315 11268 True
Enter the index of the instruction you want to fault
>>> 393 4326 11268 False
Enter the index of the instruction you want to fault
>>> 394 4337 11268 True
Enter the index of the instruction you want to fault
>>> 395 4348 11268 True
Enter the index of the instruction you want to fault
>>> 396 4359 11268 True
Enter the index of the instruction you want to fault
>>> 397 4370 11268 False
Enter the index of the instruction you want to fault
>>> 398 4381 11268 True
Enter the index of the instruction you want to fault
>>> 399 4392 11268 False
Enter the index of the instruction you want to fault
>>> 400 4403 11268 True
Enter the index of the instruction you want to fault
>>> 401 4414 11268 True
Enter the index of the instruction you want to fault
>>> 402 4425 11268 True
Enter the index of the instruction you want to fault
>>> 403 4436 11268 True
Enter the index of the instruction you want to fault
>>> 404 4447 11268 False
Enter the index of the instruction you want to fault
>>> 405 4458 11268 False
Enter the index of the instruction you want to fault
>>> 406 4469 11268 True
Enter the index of the instruction you want to fault
>>> 407 4480 11268 True
Enter the index of the instruction you want to fault
>>> 408 4491 11268 False
Enter the index of the instruction you want to fault
>>> 409 4502 11268 False
Enter the index of the instruction you want to fault
>>> 410 4513 11268 True
Enter the index of the instruction you want to fault
>>> 411 4524 11268 False
Enter the index of the instruction you want to fault
>>> 412 4535 11268 True
Enter the index of the instruction you want to fault
>>> 413 4546 11268 True
Enter the index of the instruction you want to fault
>>> 414 4557 11268 True
Enter the index of the instruction you want to fault
>>> 415 4568 11268 True
Enter the index of the instruction you want to fault
>>> 416 4579 11268 True
Enter the index of the instruction you want to fault
>>> 417 4590 11268 False
Enter the index of the instruction you want to fault
>>> 418 4601 11268 True
Enter the index of the instruction you want to fault
>>> 419 4612 11268 False
Enter the index of the instruction you want to fault
>>> 420 4623 11268 True
Enter the index of the instruction you want to fault
>>> 421 4634 11268 True
Enter the index of the instruction you want to fault
>>> 422 4645 11268 True
Enter the index of the instruction you want to fault
>>> 423 4656 11268 True
Enter the index of the instruction you want to fault
>>> 424 4667 11268 False
Enter the index of the instruction you want to fault
>>> 425 4678 11268 False
Enter the index of the instruction you want to fault
>>> 426 4689 11268 False
Enter the index of the instruction you want to fault
>>> 427 4700 11268 True
Enter the index of the instruction you want to fault
>>> 428 4711 11268 True
Enter the index of the instruction you want to fault
>>> 429 4722 11268 True
Enter the index of the instruction you want to fault
>>> 430 4733 11268 False
Enter the index of the instruction you want to fault
>>> 431 4744 11268 True
Enter the index of the instruction you want to fault
>>> 432 4755 11268 True
Enter the index of the instruction you want to fault
>>> 433 4766 11268 True
Enter the index of the instruction you want to fault
>>> 434 4777 11268 False
Enter the index of the instruction you want to fault
>>> 435 4788 11268 False
Enter the index of the instruction you want to fault
>>> 436 4799 11268 True
Enter the index of the instruction you want to fault
>>> 437 4810 11268 True
Enter the index of the instruction you want to fault
>>> 438 4821 11268 True
Enter the index of the instruction you want to fault
>>> 439 4832 11268 True
Enter the index of the instruction you want to fault
>>> 440 4843 11268 True
Enter the index of the instruction you want to fault
>>> 441 4854 11268 False
Enter the index of the instruction you want to fault
>>> 442 4865 11268 True
Enter the index of the instruction you want to fault
>>> 443 4876 11268 True
Enter the index of the instruction you want to fault
>>> 444 4887 11268 False
Enter the index of the instruction you want to fault
>>> 445 4898 11268 True
Enter the index of the instruction you want to fault
>>> 446 4909 11268 True
Enter the index of the instruction you want to fault
>>> 447 4920 11268 False
Enter the index of the instruction you want to fault
>>> 448 4931 11268 False
Enter the index of the instruction you want to fault
>>> 449 4942 11268 True
Enter the index of the instruction you want to fault
>>> 450 4953 11268 False
Enter the index of the instruction you want to fault
>>> 451 4964 11268 True
Enter the index of the instruction you want to fault
>>> 452 4975 11268 False
Enter the index of the instruction you want to fault
>>> 453 4986 11268 True
Enter the index of the instruction you want to fault
>>> 454 4997 11268 False
Enter the index of the instruction you want to fault
>>> 455 5008 11268 True
Enter the index of the instruction you want to fault
>>> 456 5019 11268 True
Enter the index of the instruction you want to fault
>>> 457 5030 11268 False
Enter the index of the instruction you want to fault
>>> 458 5041 11268 False
Enter the index of the instruction you want to fault
>>> 459 5052 11268 True
Enter the index of the instruction you want to fault
>>> 460 5063 11268 True
Enter the index of the instruction you want to fault
>>> 461 5074 11268 True
Enter the index of the instruction you want to fault
>>> 462 5085 11268 False
Enter the index of the instruction you want to fault
>>> 463 5096 11268 False
Enter the index of the instruction you want to fault
>>> 464 5107 11268 True
Enter the index of the instruction you want to fault
>>> 465 5118 11268 False
Enter the index of the instruction you want to fault
>>> 466 5129 11268 False
Enter the index of the instruction you want to fault
>>> 467 5140 11268 True
Enter the index of the instruction you want to fault
>>> 468 5151 11268 False
Enter the index of the instruction you want to fault
>>> 469 5162 11268 False
Enter the index of the instruction you want to fault
>>> 470 5173 11268 True
Enter the index of the instruction you want to fault
>>> 471 5184 11268 False
Enter the index of the instruction you want to fault
>>> 472 5195 11268 False
Enter the index of the instruction you want to fault
>>> 473 5206 11268 True
Enter the index of the instruction you want to fault
>>> 474 5217 11268 False
Enter the index of the instruction you want to fault
>>> 475 5228 11268 False
Enter the index of the instruction you want to fault
>>> 476 5239 11268 True
Enter the index of the instruction you want to fault
>>> 477 5250 11268 False
Enter the index of the instruction you want to fault
>>> 478 5261 11268 True
Enter the index of the instruction you want to fault
>>> 479 5272 11268 False
Enter the index of the instruction you want to fault
>>> 480 5283 11268 False
Enter the index of the instruction you want to fault
>>> 481 5294 11268 True
Enter the index of the instruction you want to fault
>>> 482 5305 11268 False
Enter the index of the instruction you want to fault
>>> 483 5316 11268 False
Enter the index of the instruction you want to fault
>>> 484 5327 11268 False
Enter the index of the instruction you want to fault
>>> 485 5338 11268 True
Enter the index of the instruction you want to fault
>>> 486 5349 11268 False
Enter the index of the instruction you want to fault
>>> 487 5360 11268 False
Enter the index of the instruction you want to fault
>>> 488 5371 11268 False
Enter the index of the instruction you want to fault
>>> 489 5382 11268 True
Enter the index of the instruction you want to fault
>>> 490 5393 11268 False
Enter the index of the instruction you want to fault
>>> 491 5404 11268 False
Enter the index of the instruction you want to fault
>>> 492 5415 11268 True
Enter the index of the instruction you want to fault
>>> 493 5426 11268 True
Enter the index of the instruction you want to fault
>>> 494 5437 11268 False
Enter the index of the instruction you want to fault
>>> 495 5448 11268 False
Enter the index of the instruction you want to fault
>>> 496 5459 11268 True
Enter the index of the instruction you want to fault
>>> 497 5470 11268 False
Enter the index of the instruction you want to fault
>>> 498 5481 11268 True
Enter the index of the instruction you want to fault
>>> 499 5492 11268 False
Enter the index of the instruction you want to fault
>>> 500 5503 11268 True
Enter the index of the instruction you want to fault
>>> 501 5514 11268 False
Enter the index of the instruction you want to fault
>>> 502 5525 11268 True
Enter the index of the instruction you want to fault
>>> 503 5536 11268 False
Enter the index of the instruction you want to fault
>>> 504 5547 11268 False
Enter the index of the instruction you want to fault
>>> 505 5558 11268 True
Enter the index of the instruction you want to fault
>>> 506 5569 11268 False
Enter the index of the instruction you want to fault
>>> 507 5580 11268 False
Enter the index of the instruction you want to fault
>>> 508 5591 11268 False
Enter the index of the instruction you want to fault
>>> 509 5602 11268 False
Enter the index of the instruction you want to fault
>>> 510 5613 11268 False
Enter the index of the instruction you want to fault
>>> 511 5624 11268 False
Enter the index of the instruction you want to fault
>>> 512 5635 11268 False
Enter the index of the instruction you want to fault
>>> 513 5646 11268 True
Enter the index of the instruction you want to fault
>>> 514 5657 11268 False
Enter the index of the instruction you want to fault
>>> 515 5668 11268 False
Enter the index of the instruction you want to fault
>>> 516 5679 11268 False
Enter the index of the instruction you want to fault
>>> 517 5690 11268 True
Enter the index of the instruction you want to fault
>>> 518 5701 11268 True
Enter the index of the instruction you want to fault
>>> 519 5712 11268 True
Enter the index of the instruction you want to fault
>>> 520 5723 11268 False
Enter the index of the instruction you want to fault
>>> 521 5734 11268 False
Enter the index of the instruction you want to fault
>>> 522 5745 11268 True
Enter the index of the instruction you want to fault
>>> 523 5756 11268 True
Enter the index of the instruction you want to fault
>>> 524 5767 11268 False
Enter the index of the instruction you want to fault
>>> 525 5778 11268 True
Enter the index of the instruction you want to fault
>>> 526 5789 11268 True
Enter the index of the instruction you want to fault
>>> 527 5800 11268 True
Enter the index of the instruction you want to fault
>>> 528 5811 11268 False
Enter the index of the instruction you want to fault
>>> 529 5822 11268 False
Enter the index of the instruction you want to fault
>>> 530 5833 11268 True
Enter the index of the instruction you want to fault
>>> 531 5844 11268 False
Enter the index of the instruction you want to fault
>>> 532 5855 11268 False
Enter the index of the instruction you want to fault
>>> 533 5866 11268 False
Enter the index of the instruction you want to fault
>>> 534 5877 11268 True
Enter the index of the instruction you want to fault
>>> 535 5888 11268 False
Enter the index of the instruction you want to fault
>>> 536 5899 11268 False
Enter the index of the instruction you want to fault
>>> 537 5910 11268 False
Enter the index of the instruction you want to fault
>>> 538 5921 11268 True
Enter the index of the instruction you want to fault
>>> 539 5932 11268 True
Enter the index of the instruction you want to fault
>>> 540 5943 11268 True
Enter the index of the instruction you want to fault
>>> 541 5954 11268 True
Enter the index of the instruction you want to fault
>>> 542 5965 11268 True
Enter the index of the instruction you want to fault
>>> 543 5976 11268 False
Enter the index of the instruction you want to fault
>>> 544 5987 11268 False
Enter the index of the instruction you want to fault
>>> 545 5998 11268 True
Enter the index of the instruction you want to fault
>>> 546 6009 11268 False
Enter the index of the instruction you want to fault
>>> 547 6020 11268 False
Enter the index of the instruction you want to fault
>>> 548 6031 11268 True
Enter the index of the instruction you want to fault
>>> 549 6042 11268 False
Enter the index of the instruction you want to fault
>>> 550 6053 11268 False
Enter the index of the instruction you want to fault
>>> 551 6064 11268 False
Enter the index of the instruction you want to fault
>>> 552 6075 11268 True
Enter the index of the instruction you want to fault
>>> 553 6086 11268 True
Enter the index of the instruction you want to fault
>>> 554 6097 11268 False
Enter the index of the instruction you want to fault
>>> 555 6108 11268 False
Enter the index of the instruction you want to fault
>>> 556 6119 11268 True
Enter the index of the instruction you want to fault
>>> 557 6130 11268 True
Enter the index of the instruction you want to fault
>>> 558 6141 11268 True
Enter the index of the instruction you want to fault
>>> 559 6152 11268 True
Enter the index of the instruction you want to fault
>>> 560 6163 11268 False
Enter the index of the instruction you want to fault
>>> 561 6174 11268 True
Enter the index of the instruction you want to fault
>>> 562 6185 11268 True
Enter the index of the instruction you want to fault
>>> 563 6196 11268 False
Enter the index of the instruction you want to fault
>>> 564 6207 11268 False
Enter the index of the instruction you want to fault
>>> 565 6218 11268 False
Enter the index of the instruction you want to fault
>>> 566 6229 11268 False
Enter the index of the instruction you want to fault
>>> 567 6240 11268 True
Enter the index of the instruction you want to fault
>>> 568 6251 11268 False
Enter the index of the instruction you want to fault
>>> 569 6262 11268 True
Enter the index of the instruction you want to fault
>>> 570 6273 11268 True
Enter the index of the instruction you want to fault
>>> 571 6284 11268 False
Enter the index of the instruction you want to fault
>>> 572 6295 11268 False
Enter the index of the instruction you want to fault
>>> 573 6306 11268 False
Enter the index of the instruction you want to fault
>>> 574 6317 11268 True
Enter the index of the instruction you want to fault
>>> 575 6328 11268 False
Enter the index of the instruction you want to fault
>>> 576 6339 11268 False
Enter the index of the instruction you want to fault
>>> 577 6350 11268 True
Enter the index of the instruction you want to fault
>>> 578 6361 11268 True
Enter the index of the instruction you want to fault
>>> 579 6372 11268 True
Enter the index of the instruction you want to fault
>>> 580 6383 11268 False
Enter the index of the instruction you want to fault
>>> 581 6394 11268 True
Enter the index of the instruction you want to fault
>>> 582 6405 11268 True
Enter the index of the instruction you want to fault
>>> 583 6416 11268 False
Enter the index of the instruction you want to fault
>>> 584 6427 11268 True
Enter the index of the instruction you want to fault
>>> 585 6438 11268 False
Enter the index of the instruction you want to fault
>>> 586 6449 11268 True
Enter the index of the instruction you want to fault
>>> 587 6460 11268 True
Enter the index of the instruction you want to fault
>>> 588 6471 11268 False
Enter the index of the instruction you want to fault
>>> 589 6482 11268 False
Enter the index of the instruction you want to fault
>>> 590 6493 11268 False
Enter the index of the instruction you want to fault
>>> 591 6504 11268 False
Enter the index of the instruction you want to fault
>>> 592 6515 11268 False
Enter the index of the instruction you want to fault
>>> 593 6526 11268 False
Enter the index of the instruction you want to fault
>>> 594 6537 11268 True
Enter the index of the instruction you want to fault
>>> 595 6548 11268 True
Enter the index of the instruction you want to fault
>>> 596 6559 11268 False
Enter the index of the instruction you want to fault
>>> 597 6570 11268 False
Enter the index of the instruction you want to fault
>>> 598 6581 11268 False
Enter the index of the instruction you want to fault
>>> 599 6592 11268 True
Enter the index of the instruction you want to fault
>>> 600 6603 11268 False
Enter the index of the instruction you want to fault
>>> 601 6614 11268 False
Enter the index of the instruction you want to fault
>>> 602 6625 11268 False
Enter the index of the instruction you want to fault
>>> 603 6636 11268 True
Enter the index of the instruction you want to fault
>>> 604 6647 11268 True
Enter the index of the instruction you want to fault
>>> 605 6658 11268 True
Enter the index of the instruction you want to fault
>>> 606 6669 11268 True
Enter the index of the instruction you want to fault
>>> 607 6680 11268 True
Enter the index of the instruction you want to fault
>>> 608 6691 11268 True
Enter the index of the instruction you want to fault
>>> 609 6702 11268 False
Enter the index of the instruction you want to fault
>>> 610 6713 11268 False
Enter the index of the instruction you want to fault
>>> 611 6724 11268 True
Enter the index of the instruction you want to fault
>>> 612 6735 11268 True
Enter the index of the instruction you want to fault
>>> 613 6746 11268 True
Enter the index of the instruction you want to fault
>>> 614 6757 11268 True
Enter the index of the instruction you want to fault
>>> 615 6768 11268 False
Enter the index of the instruction you want to fault
>>> 616 6779 11268 True
Enter the index of the instruction you want to fault
>>> 617 6790 11268 False
Enter the index of the instruction you want to fault
>>> 618 6801 11268 False
Enter the index of the instruction you want to fault
>>> 619 6812 11268 False
Enter the index of the instruction you want to fault
>>> 620 6823 11268 True
Enter the index of the instruction you want to fault
>>> 621 6834 11268 False
Enter the index of the instruction you want to fault
>>> 622 6845 11268 False
Enter the index of the instruction you want to fault
>>> 623 6856 11268 True
Enter the index of the instruction you want to fault
>>> 624 6867 11268 True
Enter the index of the instruction you want to fault
>>> 625 6878 11268 True
Enter the index of the instruction you want to fault
>>> 626 6889 11268 True
Enter the index of the instruction you want to fault
>>> 627 6900 11268 True
Enter the index of the instruction you want to fault
>>> 628 6911 11268 False
Enter the index of the instruction you want to fault
>>> 629 6922 11268 True
Enter the index of the instruction you want to fault
>>> 630 6933 11268 True
Enter the index of the instruction you want to fault
>>> 631 6944 11268 False
Enter the index of the instruction you want to fault
>>> 632 6955 11268 False
Enter the index of the instruction you want to fault
>>> 633 6966 11268 False
Enter the index of the instruction you want to fault
>>> 634 6977 11268 False
Enter the index of the instruction you want to fault
>>> 635 6988 11268 True
Enter the index of the instruction you want to fault
>>> 636 6999 11268 False
Enter the index of the instruction you want to fault
>>> 637 7010 11268 True
Enter the index of the instruction you want to fault
>>> 638 7021 11268 False
Enter the index of the instruction you want to fault
>>> 639 7032 11268 False
Enter the index of the instruction you want to fault
>>> 640 7043 11268 False
Enter the index of the instruction you want to fault
>>> 641 7054 11268 True
Enter the index of the instruction you want to fault
>>> 642 7065 11268 True
Enter the index of the instruction you want to fault
>>> 643 7076 11268 True
Enter the index of the instruction you want to fault
>>> 644 7087 11268 False
Enter the index of the instruction you want to fault
>>> 645 7098 11268 False
Enter the index of the instruction you want to fault
>>> 646 7109 11268 False
Enter the index of the instruction you want to fault
>>> 647 7120 11268 False
Enter the index of the instruction you want to fault
>>> 648 7131 11268 True
Enter the index of the instruction you want to fault
>>> 649 7142 11268 True
Enter the index of the instruction you want to fault
>>> 650 7153 11268 True
Enter the index of the instruction you want to fault
>>> 651 7164 11268 False
Enter the index of the instruction you want to fault
>>> 652 7175 11268 False
Enter the index of the instruction you want to fault
>>> 653 7186 11268 True
Enter the index of the instruction you want to fault
>>> 654 7197 11268 False
Enter the index of the instruction you want to fault
>>> 655 7208 11268 False
Enter the index of the instruction you want to fault
>>> 656 7219 11268 False
Enter the index of the instruction you want to fault
>>> 657 7230 11268 False
Enter the index of the instruction you want to fault
>>> 658 7241 11268 True
Enter the index of the instruction you want to fault
>>> 659 7252 11268 True
Enter the index of the instruction you want to fault
>>> 660 7263 11268 True
Enter the index of the instruction you want to fault
>>> 661 7274 11268 False
Enter the index of the instruction you want to fault
>>> 662 7285 11268 True
Enter the index of the instruction you want to fault
>>> 663 7296 11268 True
Enter the index of the instruction you want to fault
>>> 664 7307 11268 True
Enter the index of the instruction you want to fault
>>> 665 7318 11268 False
Enter the index of the instruction you want to fault
>>> 666 7329 11268 False
Enter the index of the instruction you want to fault
>>> 667 7340 11268 False
Enter the index of the instruction you want to fault
>>> 668 7351 11268 True
Enter the index of the instruction you want to fault
>>> 669 7362 11268 False
Enter the index of the instruction you want to fault
>>> 670 7373 11268 False
Enter the index of the instruction you want to fault
>>> 671 7384 11268 True
Enter the index of the instruction you want to fault
>>> 672 7395 11268 True
Enter the index of the instruction you want to fault
>>> 673 7406 11268 True
Enter the index of the instruction you want to fault
>>> 674 7417 11268 False
Enter the index of the instruction you want to fault
>>> 675 7428 11268 False
Enter the index of the instruction you want to fault
>>> 676 7439 11268 False
Enter the index of the instruction you want to fault
>>> 677 7450 11268 True
Enter the index of the instruction you want to fault
>>> 678 7461 11268 False
Enter the index of the instruction you want to fault
>>> 679 7472 11268 True
Enter the index of the instruction you want to fault
>>> 680 7483 11268 False
Enter the index of the instruction you want to fault
>>> 681 7494 11268 False
Enter the index of the instruction you want to fault
>>> 682 7505 11268 False
Enter the index of the instruction you want to fault
>>> 683 7516 11268 False
Enter the index of the instruction you want to fault
>>> 684 7527 11268 True
Enter the index of the instruction you want to fault
>>> 685 7538 11268 False
Enter the index of the instruction you want to fault
>>> 686 7549 11268 False
Enter the index of the instruction you want to fault
>>> 687 7560 11268 False
Enter the index of the instruction you want to fault
>>> 688 7571 11268 False
Enter the index of the instruction you want to fault
>>> 689 7582 11268 False
Enter the index of the instruction you want to fault
>>> 690 7593 11268 False
Enter the index of the instruction you want to fault
>>> 691 7604 11268 False
Enter the index of the instruction you want to fault
>>> 692 7615 11268 True
Enter the index of the instruction you want to fault
>>> 693 7626 11268 False
Enter the index of the instruction you want to fault
>>> 694 7637 11268 True
Enter the index of the instruction you want to fault
>>> 695 7648 11268 False
Enter the index of the instruction you want to fault
>>> 696 7659 11268 False
Enter the index of the instruction you want to fault
>>> 697 7670 11268 True
Enter the index of the instruction you want to fault
>>> 698 7681 11268 True
Enter the index of the instruction you want to fault
>>> 699 7692 11268 True
Enter the index of the instruction you want to fault
>>> 700 7703 11268 False
Enter the index of the instruction you want to fault
>>> 701 7714 11268 True
Enter the index of the instruction you want to fault
>>> 702 7725 11268 False
Enter the index of the instruction you want to fault
>>> 703 7736 11268 False
Enter the index of the instruction you want to fault
>>> 704 7747 11268 False
Enter the index of the instruction you want to fault
>>> 705 7758 11268 False
Enter the index of the instruction you want to fault
>>> 706 7769 11268 True
Enter the index of the instruction you want to fault
>>> 707 7780 11268 True
Enter the index of the instruction you want to fault
>>> 708 7791 11268 True
Enter the index of the instruction you want to fault
>>> 709 7802 11268 True
Enter the index of the instruction you want to fault
>>> 710 7813 11268 True
Enter the index of the instruction you want to fault
>>> 711 7824 11268 False
Enter the index of the instruction you want to fault
>>> 712 7835 11268 False
Enter the index of the instruction you want to fault
>>> 713 7846 11268 False
Enter the index of the instruction you want to fault
>>> 714 7857 11268 True
Enter the index of the instruction you want to fault
>>> 715 7868 11268 True
Enter the index of the instruction you want to fault
>>> 716 7879 11268 True
Enter the index of the instruction you want to fault
>>> 717 7890 11268 False
Enter the index of the instruction you want to fault
>>> 718 7901 11268 False
Enter the index of the instruction you want to fault
>>> 719 7912 11268 False
Enter the index of the instruction you want to fault
>>> 720 7923 11268 True
Enter the index of the instruction you want to fault
>>> 721 7934 11268 True
Enter the index of the instruction you want to fault
>>> 722 7945 11268 True
Enter the index of the instruction you want to fault
>>> 723 7956 11268 False
Enter the index of the instruction you want to fault
>>> 724 7967 11268 False
Enter the index of the instruction you want to fault
>>> 725 7978 11268 False
Enter the index of the instruction you want to fault
>>> 726 7989 11268 True
Enter the index of the instruction you want to fault
>>> 727 8000 11268 False
Enter the index of the instruction you want to fault
>>> 728 8011 11268 True
Enter the index of the instruction you want to fault
>>> 729 8022 11268 False
Enter the index of the instruction you want to fault
>>> 730 8033 11268 True
Enter the index of the instruction you want to fault
>>> 731 8044 11268 False
Enter the index of the instruction you want to fault
>>> 732 8055 11268 True
Enter the index of the instruction you want to fault
>>> 733 8066 11268 True
Enter the index of the instruction you want to fault
>>> 734 8077 11268 True
Enter the index of the instruction you want to fault
>>> 735 8088 11268 True
Enter the index of the instruction you want to fault
>>> 736 8099 11268 False
Enter the index of the instruction you want to fault
>>> 737 8110 11268 True
Enter the index of the instruction you want to fault
>>> 738 8121 11268 False
Enter the index of the instruction you want to fault
>>> 739 8132 11268 True
Enter the index of the instruction you want to fault
>>> 740 8143 11268 False
Enter the index of the instruction you want to fault
>>> 741 8154 11268 False
Enter the index of the instruction you want to fault
>>> 742 8165 11268 False
Enter the index of the instruction you want to fault
>>> 743 8176 11268 True
Enter the index of the instruction you want to fault
>>> 744 8187 11268 True
Enter the index of the instruction you want to fault
>>> 745 8198 11268 False
Enter the index of the instruction you want to fault
>>> 746 8209 11268 False
Enter the index of the instruction you want to fault
>>> 747 8220 11268 False
Enter the index of the instruction you want to fault
>>> 748 8231 11268 True
Enter the index of the instruction you want to fault
>>> 749 8242 11268 True
Enter the index of the instruction you want to fault
>>> 750 8253 11268 False
Enter the index of the instruction you want to fault
>>> 751 8264 11268 True
Enter the index of the instruction you want to fault
>>> 752 8275 11268 True
Enter the index of the instruction you want to fault
>>> 753 8286 11268 True
Enter the index of the instruction you want to fault
>>> 754 8297 11268 True
Enter the index of the instruction you want to fault
>>> 755 8308 11268 False
Enter the index of the instruction you want to fault
>>> 756 8319 11268 False
Enter the index of the instruction you want to fault
>>> 757 8330 11268 True
Enter the index of the instruction you want to fault
>>> 758 8341 11268 True
Enter the index of the instruction you want to fault
>>> 759 8352 11268 False
Enter the index of the instruction you want to fault
>>> 760 8363 11268 False
Enter the index of the instruction you want to fault
>>> 761 8374 11268 True
Enter the index of the instruction you want to fault
>>> 762 8385 11268 False
Enter the index of the instruction you want to fault
>>> 763 8396 11268 True
Enter the index of the instruction you want to fault
>>> 764 8407 11268 False
Enter the index of the instruction you want to fault
>>> 765 8418 11268 True
Enter the index of the instruction you want to fault
>>> 766 8429 11268 True
Enter the index of the instruction you want to fault
>>> 767 8440 11268 False
Enter the index of the instruction you want to fault
>>> 768 8451 11268 False
Enter the index of the instruction you want to fault
>>> 769 8462 11268 False
Enter the index of the instruction you want to fault
>>> 770 8473 11268 True
Enter the index of the instruction you want to fault
>>> 771 8484 11268 True
Enter the index of the instruction you want to fault
>>> 772 8495 11268 True
Enter the index of the instruction you want to fault
>>> 773 8506 11268 True
Enter the index of the instruction you want to fault
>>> 774 8517 11268 False
Enter the index of the instruction you want to fault
>>> 775 8528 11268 False
Enter the index of the instruction you want to fault
>>> 776 8539 11268 True
Enter the index of the instruction you want to fault
>>> 777 8550 11268 True
Enter the index of the instruction you want to fault
>>> 778 8561 11268 False
Enter the index of the instruction you want to fault
>>> 779 8572 11268 False
Enter the index of the instruction you want to fault
>>> 780 8583 11268 True
Enter the index of the instruction you want to fault
>>> 781 8594 11268 True
Enter the index of the instruction you want to fault
>>> 782 8605 11268 True
Enter the index of the instruction you want to fault
>>> 783 8616 11268 False
Enter the index of the instruction you want to fault
>>> 784 8627 11268 False
Enter the index of the instruction you want to fault
>>> 785 8638 11268 True
Enter the index of the instruction you want to fault
>>> 786 8649 11268 True
Enter the index of the instruction you want to fault
>>> 787 8660 11268 False
Enter the index of the instruction you want to fault
>>> 788 8671 11268 False
Enter the index of the instruction you want to fault
>>> 789 8682 11268 True
Enter the index of the instruction you want to fault
>>> 790 8693 11268 False
Enter the index of the instruction you want to fault
>>> 791 8704 11268 True
Enter the index of the instruction you want to fault
>>> 792 8715 11268 False
Enter the index of the instruction you want to fault
>>> 793 8726 11268 False
Enter the index of the instruction you want to fault
>>> 794 8737 11268 False
Enter the index of the instruction you want to fault
>>> 795 8748 11268 True
Enter the index of the instruction you want to fault
>>> 796 8759 11268 True
Enter the index of the instruction you want to fault
>>> 797 8770 11268 False
Enter the index of the instruction you want to fault
>>> 798 8781 11268 False
Enter the index of the instruction you want to fault
>>> 799 8792 11268 False
Enter the index of the instruction you want to fault
>>> 800 8803 11268 False
Enter the index of the instruction you want to fault
>>> 801 8814 11268 True
Enter the index of the instruction you want to fault
>>> 802 8825 11268 False
Enter the index of the instruction you want to fault
>>> 803 8836 11268 True
Enter the index of the instruction you want to fault
>>> 804 8847 11268 True
Enter the index of the instruction you want to fault
>>> 805 8858 11268 False
Enter the index of the instruction you want to fault
>>> 806 8869 11268 True
Enter the index of the instruction you want to fault
>>> 807 8880 11268 False
Enter the index of the instruction you want to fault
>>> 808 8891 11268 True
Enter the index of the instruction you want to fault
>>> 809 8902 11268 False
Enter the index of the instruction you want to fault
>>> 810 8913 11268 False
Enter the index of the instruction you want to fault
>>> 811 8924 11268 False
Enter the index of the instruction you want to fault
>>> 812 8935 11268 True
Enter the index of the instruction you want to fault
>>> 813 8946 11268 False
Enter the index of the instruction you want to fault
>>> 814 8957 11268 True
Enter the index of the instruction you want to fault
>>> 815 8968 11268 False
Enter the index of the instruction you want to fault
>>> 816 8979 11268 True
Enter the index of the instruction you want to fault
>>> 817 8990 11268 True
Enter the index of the instruction you want to fault
>>> 818 9001 11268 False
Enter the index of the instruction you want to fault
>>> 819 9012 11268 False
Enter the index of the instruction you want to fault
>>> 820 9023 11268 False
Enter the index of the instruction you want to fault
>>> 821 9034 11268 True
Enter the index of the instruction you want to fault
>>> 822 9045 11268 True
Enter the index of the instruction you want to fault
>>> 823 9056 11268 True
Enter the index of the instruction you want to fault
>>> 824 9067 11268 True
Enter the index of the instruction you want to fault
>>> 825 9078 11268 False
Enter the index of the instruction you want to fault
>>> 826 9089 11268 True
Enter the index of the instruction you want to fault
>>> 827 9100 11268 True
Enter the index of the instruction you want to fault
>>> 828 9111 11268 False
Enter the index of the instruction you want to fault
>>> 829 9122 11268 True
Enter the index of the instruction you want to fault
>>> 830 9133 11268 True
Enter the index of the instruction you want to fault
>>> 831 9144 11268 True
Enter the index of the instruction you want to fault
>>> 832 9155 11268 False
Enter the index of the instruction you want to fault
>>> 833 9166 11268 True
Enter the index of the instruction you want to fault
>>> 834 9177 11268 True
Enter the index of the instruction you want to fault
>>> 835 9188 11268 False
Enter the index of the instruction you want to fault
>>> 836 9199 11268 True
Enter the index of the instruction you want to fault
>>> 837 9210 11268 True
Enter the index of the instruction you want to fault
>>> 838 9221 11268 False
Enter the index of the instruction you want to fault
>>> 839 9232 11268 False
Enter the index of the instruction you want to fault
>>> 840 9243 11268 True
Enter the index of the instruction you want to fault
>>> 841 9254 11268 False
Enter the index of the instruction you want to fault
>>> 842 9265 11268 True
Enter the index of the instruction you want to fault
>>> 843 9276 11268 True
Enter the index of the instruction you want to fault
>>> 844 9287 11268 True
Enter the index of the instruction you want to fault
>>> 845 9298 11268 False
Enter the index of the instruction you want to fault
>>> 846 9309 11268 False
Enter the index of the instruction you want to fault
>>> 847 9320 11268 True
Enter the index of the instruction you want to fault
>>> 848 9331 11268 False
Enter the index of the instruction you want to fault
>>> 849 9342 11268 False
Enter the index of the instruction you want to fault
>>> 850 9353 11268 True
Enter the index of the instruction you want to fault
>>> 851 9364 11268 False
Enter the index of the instruction you want to fault
>>> 852 9375 11268 False
Enter the index of the instruction you want to fault
>>> 853 9386 11268 True
Enter the index of the instruction you want to fault
>>> 854 9397 11268 True
Enter the index of the instruction you want to fault
>>> 855 9408 11268 False
Enter the index of the instruction you want to fault
>>> 856 9419 11268 True
Enter the index of the instruction you want to fault
>>> 857 9430 11268 True
Enter the index of the instruction you want to fault
>>> 858 9441 11268 False
Enter the index of the instruction you want to fault
>>> 859 9452 11268 False
Enter the index of the instruction you want to fault
>>> 860 9463 11268 True
Enter the index of the instruction you want to fault
>>> 861 9474 11268 True
Enter the index of the instruction you want to fault
>>> 862 9485 11268 False
Enter the index of the instruction you want to fault
>>> 863 9496 11268 False
Enter the index of the instruction you want to fault
>>> 864 9507 11268 False
Enter the index of the instruction you want to fault
>>> 865 9518 11268 False
Enter the index of the instruction you want to fault
>>> 866 9529 11268 False
Enter the index of the instruction you want to fault
>>> 867 9540 11268 False
Enter the index of the instruction you want to fault
>>> 868 9551 11268 True
Enter the index of the instruction you want to fault
>>> 869 9562 11268 False
Enter the index of the instruction you want to fault
>>> 870 9573 11268 True
Enter the index of the instruction you want to fault
>>> 871 9584 11268 False
Enter the index of the instruction you want to fault
>>> 872 9595 11268 False
Enter the index of the instruction you want to fault
>>> 873 9606 11268 False
Enter the index of the instruction you want to fault
>>> 874 9617 11268 True
Enter the index of the instruction you want to fault
>>> 875 9628 11268 False
Enter the index of the instruction you want to fault
>>> 876 9639 11268 False
Enter the index of the instruction you want to fault
>>> 877 9650 11268 True
Enter the index of the instruction you want to fault
>>> 878 9661 11268 True
Enter the index of the instruction you want to fault
>>> 879 9672 11268 True
Enter the index of the instruction you want to fault
>>> 880 9683 11268 True
Enter the index of the instruction you want to fault
>>> 881 9694 11268 False
Enter the index of the instruction you want to fault
>>> 882 9705 11268 True
Enter the index of the instruction you want to fault
>>> 883 9716 11268 True
Enter the index of the instruction you want to fault
>>> 884 9727 11268 False
Enter the index of the instruction you want to fault
>>> 885 9738 11268 False
Enter the index of the instruction you want to fault
>>> 886 9749 11268 False
Enter the index of the instruction you want to fault
>>> 887 9760 11268 True
Enter the index of the instruction you want to fault
>>> 888 9771 11268 False
Enter the index of the instruction you want to fault
>>> 889 9782 11268 True
Enter the index of the instruction you want to fault
>>> 890 9793 11268 False
Enter the index of the instruction you want to fault
>>> 891 9804 11268 False
Enter the index of the instruction you want to fault
>>> 892 9815 11268 False
Enter the index of the instruction you want to fault
>>> 893 9826 11268 True
Enter the index of the instruction you want to fault
>>> 894 9837 11268 True
Enter the index of the instruction you want to fault
>>> 895 9848 11268 True
Enter the index of the instruction you want to fault
>>> 896 9859 11268 False
Enter the index of the instruction you want to fault
>>> 897 9870 11268 False
Enter the index of the instruction you want to fault
>>> 898 9881 11268 False
Enter the index of the instruction you want to fault
>>> 899 9892 11268 True
Enter the index of the instruction you want to fault
>>> 900 9903 11268 True
Enter the index of the instruction you want to fault
>>> 901 9914 11268 False
Enter the index of the instruction you want to fault
>>> 902 9925 11268 True
Enter the index of the instruction you want to fault
>>> 903 9936 11268 False
Enter the index of the instruction you want to fault
>>> 904 9947 11268 False
Enter the index of the instruction you want to fault
>>> 905 9958 11268 True
Enter the index of the instruction you want to fault
>>> 906 9969 11268 False
Enter the index of the instruction you want to fault
>>> 907 9980 11268 True
Enter the index of the instruction you want to fault
>>> 908 9991 11268 True
Enter the index of the instruction you want to fault
>>> 909 10002 11268 True
Enter the index of the instruction you want to fault
>>> 910 10013 11268 True
Enter the index of the instruction you want to fault
>>> 911 10024 11268 False
Enter the index of the instruction you want to fault
>>> 912 10035 11268 True
Enter the index of the instruction you want to fault
>>> 913 10046 11268 True
Enter the index of the instruction you want to fault
>>> 914 10057 11268 True
Enter the index of the instruction you want to fault
>>> 915 10068 11268 True
Enter the index of the instruction you want to fault
>>> 916 10079 11268 False
Enter the index of the instruction you want to fault
>>> 917 10090 11268 True
Enter the index of the instruction you want to fault
>>> 918 10101 11268 True
Enter the index of the instruction you want to fault
>>> 919 10112 11268 True
Enter the index of the instruction you want to fault
>>> 920 10123 11268 False
Enter the index of the instruction you want to fault
>>> 921 10134 11268 False
Enter the index of the instruction you want to fault
>>> 922 10145 11268 False
Enter the index of the instruction you want to fault
>>> 923 10156 11268 False
Enter the index of the instruction you want to fault
>>> 924 10167 11268 False
Enter the index of the instruction you want to fault
>>> 925 10178 11268 False
Enter the index of the instruction you want to fault
>>> 926 10189 11268 False
Enter the index of the instruction you want to fault
>>> 927 10200 11268 True
Enter the index of the instruction you want to fault
>>> 928 10211 11268 False
Enter the index of the instruction you want to fault
>>> 929 10222 11268 True
Enter the index of the instruction you want to fault
>>> 930 10233 11268 False
Enter the index of the instruction you want to fault
>>> 931 10244 11268 False
Enter the index of the instruction you want to fault
>>> 932 10255 11268 True
Enter the index of the instruction you want to fault
>>> 933 10266 11268 True
Enter the index of the instruction you want to fault
>>> 934 10277 11268 True
Enter the index of the instruction you want to fault
>>> 935 10288 11268 False
Enter the index of the instruction you want to fault
>>> 936 10299 11268 False
Enter the index of the instruction you want to fault
>>> 937 10310 11268 False
Enter the index of the instruction you want to fault
>>> 938 10321 11268 True
Enter the index of the instruction you want to fault
>>> 939 10332 11268 False
Enter the index of the instruction you want to fault
>>> 940 10343 11268 True
Enter the index of the instruction you want to fault
>>> 941 10354 11268 False
Enter the index of the instruction you want to fault
>>> 942 10365 11268 False
Enter the index of the instruction you want to fault
>>> 943 10376 11268 True
Enter the index of the instruction you want to fault
>>> 944 10387 11268 False
Enter the index of the instruction you want to fault
>>> 945 10398 11268 True
Enter the index of the instruction you want to fault
>>> 946 10409 11268 True
Enter the index of the instruction you want to fault
>>> 947 10420 11268 False
Enter the index of the instruction you want to fault
>>> 948 10431 11268 True
Enter the index of the instruction you want to fault
>>> 949 10442 11268 False
Enter the index of the instruction you want to fault
>>> 950 10453 11268 False
Enter the index of the instruction you want to fault
>>> 951 10464 11268 True
Enter the index of the instruction you want to fault
>>> 952 10475 11268 True
Enter the index of the instruction you want to fault
>>> 953 10486 11268 False
Enter the index of the instruction you want to fault
>>> 954 10497 11268 True
Enter the index of the instruction you want to fault
>>> 955 10508 11268 False
Enter the index of the instruction you want to fault
>>> 956 10519 11268 False
Enter the index of the instruction you want to fault
>>> 957 10530 11268 False
Enter the index of the instruction you want to fault
>>> 958 10541 11268 True
Enter the index of the instruction you want to fault
>>> 959 10552 11268 True
Enter the index of the instruction you want to fault
>>> 960 10563 11268 True
Enter the index of the instruction you want to fault
>>> 961 10574 11268 False
Enter the index of the instruction you want to fault
>>> 962 10585 11268 True
Enter the index of the instruction you want to fault
>>> 963 10596 11268 True
Enter the index of the instruction you want to fault
>>> 964 10607 11268 False
Enter the index of the instruction you want to fault
>>> 965 10618 11268 False
Enter the index of the instruction you want to fault
>>> 966 10629 11268 True
Enter the index of the instruction you want to fault
>>> 967 10640 11268 False
Enter the index of the instruction you want to fault
>>> 968 10651 11268 False
Enter the index of the instruction you want to fault
>>> 969 10662 11268 True
Enter the index of the instruction you want to fault
>>> 970 10673 11268 False
Enter the index of the instruction you want to fault
>>> 971 10684 11268 True
Enter the index of the instruction you want to fault
>>> 972 10695 11268 False
Enter the index of the instruction you want to fault
>>> 973 10706 11268 True
Enter the index of the instruction you want to fault
>>> 974 10717 11268 True
Enter the index of the instruction you want to fault
>>> 975 10728 11268 True
Enter the index of the instruction you want to fault
>>> 976 10739 11268 False
Enter the index of the instruction you want to fault
>>> 977 10750 11268 True
Enter the index of the instruction you want to fault
>>> 978 10761 11268 False
Enter the index of the instruction you want to fault
>>> 979 10772 11268 True
Enter the index of the instruction you want to fault
>>> 980 10783 11268 False
Enter the index of the instruction you want to fault
>>> 981 10794 11268 True
Enter the index of the instruction you want to fault
>>> 982 10805 11268 True
Enter the index of the instruction you want to fault
>>> 983 10816 11268 False
Enter the index of the instruction you want to fault
>>> 984 10827 11268 True
Enter the index of the instruction you want to fault
>>> 985 10838 11268 False
Enter the index of the instruction you want to fault
>>> 986 10849 11268 True
Enter the index of the instruction you want to fault
>>> 987 10860 11268 False
Enter the index of the instruction you want to fault
>>> 988 10871 11268 False
Enter the index of the instruction you want to fault
>>> 989 10882 11268 False
Enter the index of the instruction you want to fault
>>> 990 10893 11268 True
Enter the index of the instruction you want to fault
>>> 991 10904 11268 False
Enter the index of the instruction you want to fault
>>> 992 10915 11268 True
Enter the index of the instruction you want to fault
>>> 993 10926 11268 True
Enter the index of the instruction you want to fault
>>> 994 10937 11268 False
Enter the index of the instruction you want to fault
>>> 995 10948 11268 True
Enter the index of the instruction you want to fault
>>> 996 10959 11268 True
Enter the index of the instruction you want to fault
>>> 997 10970 11268 False
Enter the index of the instruction you want to fault
>>> 998 10981 11268 True
Enter the index of the instruction you want to fault
>>> 999 10992 11268 True
Enter the index of the instruction you want to fault
>>> 1000 11003 11268 True
Enter the index of the instruction you want to fault
>>> 1001 11014 11268 True
Enter the index of the instruction you want to fault
>>> 1002 11025 11268 False
Enter the index of the instruction you want to fault
>>> 1003 11036 11268 False
Enter the index of the instruction you want to fault
>>> 1004 11047 11268 True
Enter the index of the instruction you want to fault
>>> 1005 11058 11268 False
Enter the index of the instruction you want to fault
>>> 1006 11069 11268 True
Enter the index of the instruction you want to fault
>>> 1007 11080 11268 False
Enter the index of the instruction you want to fault
>>> 1008 11091 11268 True
Enter the index of the instruction you want to fault
>>> 1009 11102 11268 False
Enter the index of the instruction you want to fault
>>> 1010 11113 11268 False
Enter the index of the instruction you want to fault
>>> 1011 11124 11268 False
Enter the index of the instruction you want to fault
>>> 1012 11135 11268 False
Enter the index of the instruction you want to fault
>>> 1013 11146 11268 True
Enter the index of the instruction you want to fault
>>> 1014 11157 11268 False
Enter the index of the instruction you want to fault
>>> 1015 11168 11268 False
Enter the index of the instruction you want to fault
>>> 1016 11179 11268 False
Enter the index of the instruction you want to fault
>>> 1017 11190 11268 True
Enter the index of the instruction you want to fault
>>> 1018 11201 11268 False
Enter the index of the instruction you want to fault
>>> 1019 11212 11268 True
Enter the index of the instruction you want to fault
>>> 1020 11223 11268 False
Enter the index of the instruction you want to fault
>>> 1021 11234 11268 True
Enter the index of the instruction you want to fault
>>> 1022 11245 11268 True
Enter the index of the instruction you want to fault
>>> 1023 11256 11268 False
RSA.asm
MOV R0, #0
MOV R1, #1
MOV R2, R1 ;initialize output with 1
loop:
MUL R4, R2, R6 ;current_result * A (multiply)
MOD R4, R4
AND R3, R5, R1 ;read lsb of exponent
MUL R4, R3, R4 ;R4 = current_result * A if lsb is 1
XOR R3, R3, R1
MUL R2, R3, R2 ;R2 = current_result if lsb is 0
ADD R2, R2, R4
POW R6, R6 ;A**2 (square)
SRL R5, R5, R1 ;drop lsb of exponent
CMP R5, R0 ;is exponent > 0 ?
JNZR loop
STP